Overview of the checksum example, Implementing the checksum function – Echelon Neuron User Manual
Page 66
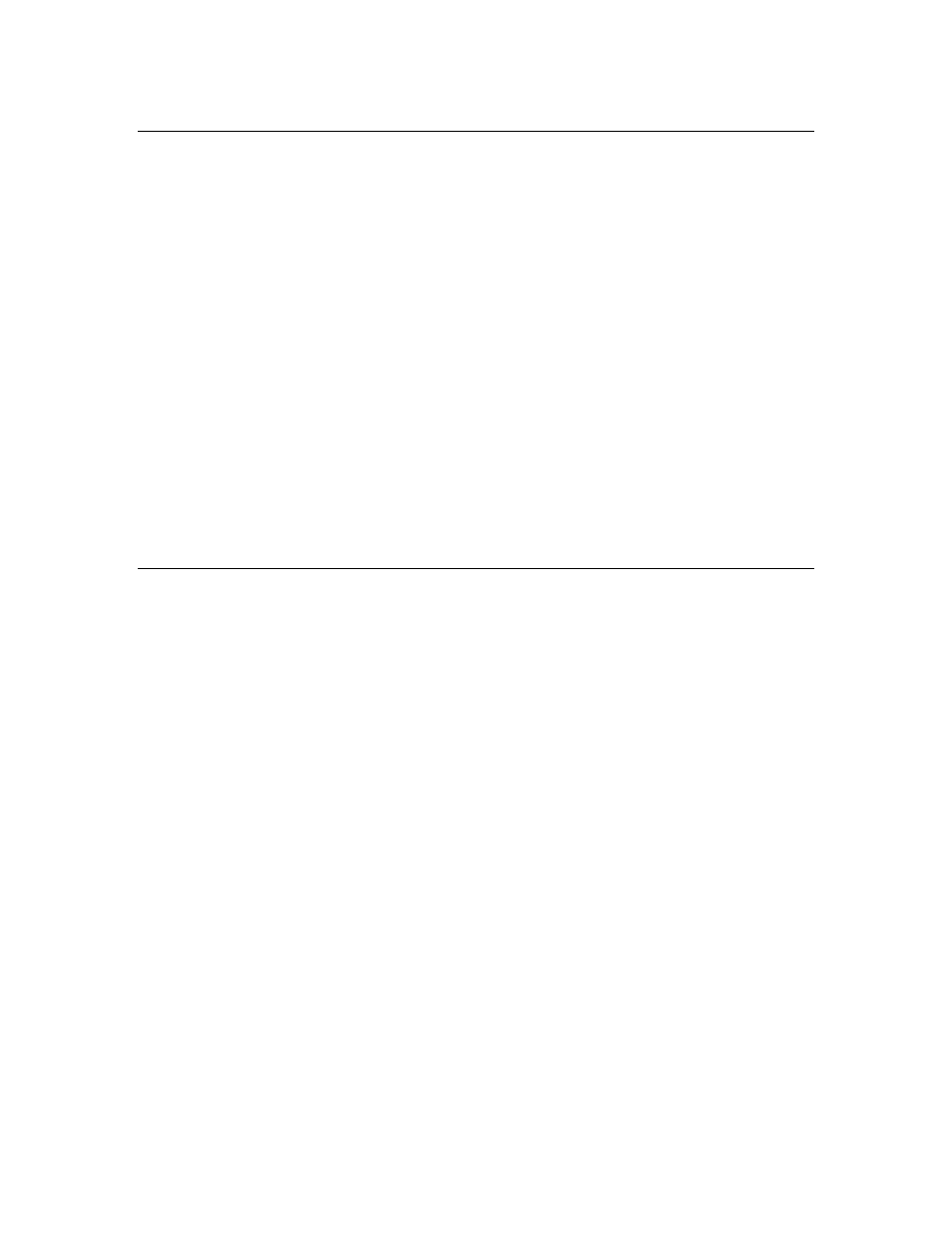
Overview of the Checksum Example
The checksum example function described in this chapter demonstrates the
process of writing and testing a function in Neuron assembly language.
The example function calculates a simple checksum for a data string of a
specified length. The checksum algorithm used combines all bytes with an
exclusive OR. An equivalent Neuron C function for the checksum example is
shown below:
unsigned Checksum(unsigned size, const char* pData) {
unsigned result;
result = 0;
while (size--) {
result ^= *pData++;
}
return result;
}
Although there might not be a need to recode this particular function in assembly
language, this example demonstrates how to pass arguments from a Neuron C
program to a Neuron Assembler function, how to manage the stacks to process a
simple calculation, and how to return arguments from a Neuron Assembler
function to a Neuron C program.
Implementing the Checksum Function
A Neuron assembly implementation of the checksum example function is shown
below:
SEG CODE
ORG
pData EQU 0
%Checksum APEXP ; (size, pData(2) -- cs)
poppush ; (pData(2)) R(size)
popd [pData] ; () R(size)
pushs #0 ; (cs) R(size)
cs_loop
push [pData][0] ; (v, cs) R(size)
inc [pData] ; (v, cs) R(size)
xor ; (cs) R(size)
dbrnz cs_loop ; (cs) R({size})
ret ; (cs) R()
The Neuron assembly code for the Checksum function performs the following
tasks:
•
The EQU directive defines a mnemonic (pData) with value 0 (zero). This
mnemonic is used to identify one of the general-purpose pointer registers
(P0). This example uses a mnemonic name (pData) that is more
meaningful to this source code than the register name (P0).
•
The POPPUSH instruction takes the value in TOS (the size argument)
from the data stack and pushes it on the return stack. TOS now has the
56
Exploring an Example Function in Neuron Assembly