Programmatic transaction management, Using the transactiontemplate, Using a platformtransactionmanager – HP Integrity NonStop H-Series User Manual
Page 84: Using the, Transactiontemplate, Using a, Platformtransactionmanager
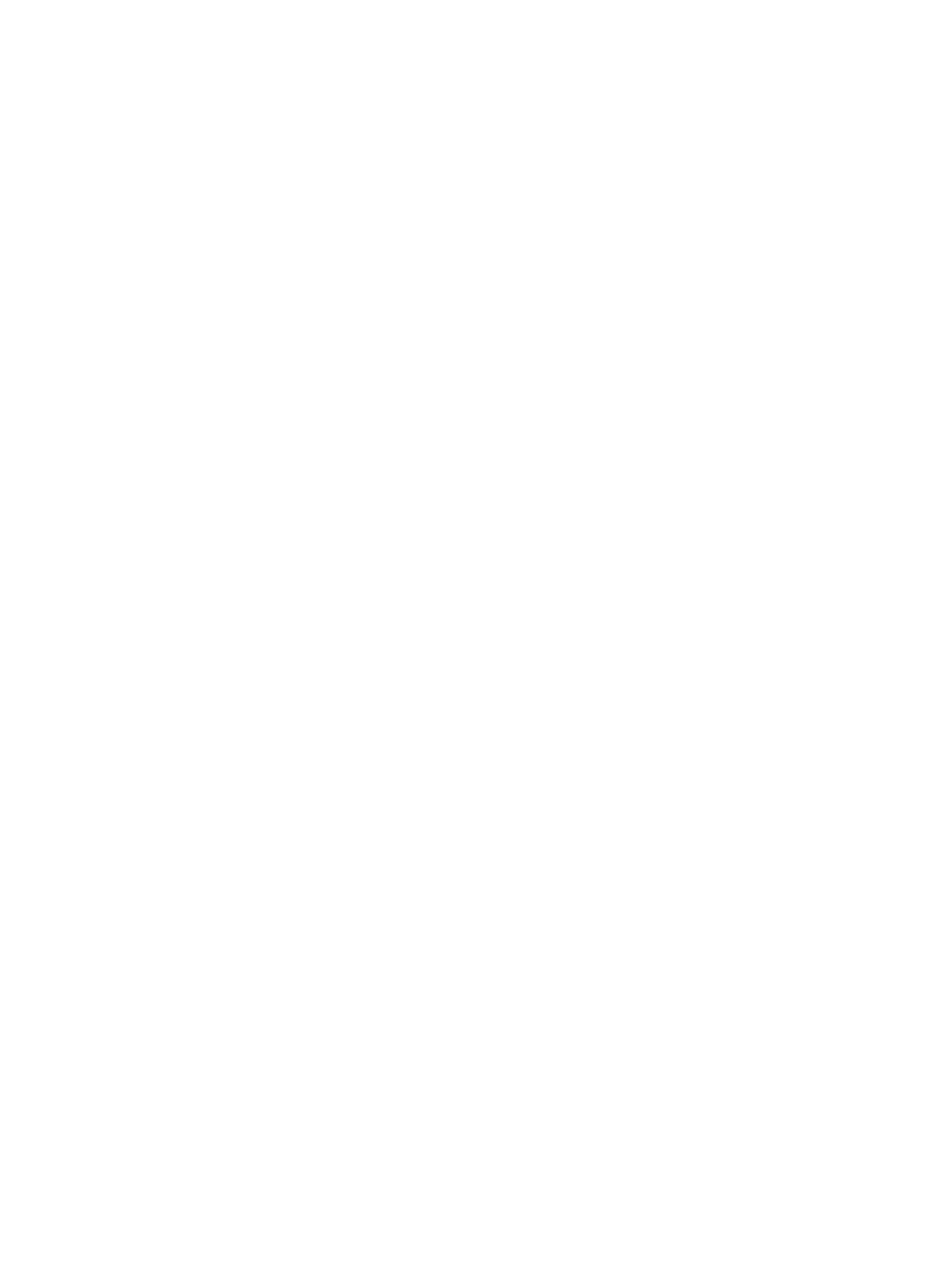
Programmatic Transaction Management
The Spring framework provides two means of programmatic transaction management:
•
•
Using the
TransactionTemplate
The
TransactionTemplate
handles the transaction lifecycle and possible exceptions so that
the calling code does not explicitly handle transactions.
The application code that must execute in a transactional context, and that uses
TransactionTemplate
explicitly, can be achieved by completing the following steps:
1.
Write a
TransactionCallback
implementation that contains all the codes you need to
execute in the context of a transaction.
2.
Pass an instance of your custom
TransactionCallback
to the
execute(..)
method
exposed on the
TransactionTemplate
.
public class SimpleService implements Service {
// single TransactionTemplate shared amongst all methods in this instance
private final TransactionTemplate transactionTemplate;
// use constructor-injection to supply the PlatformTransactionManager
public SimpleService(PlatformTransactionManager transactionManager) {
Assert.notNull(transactionManager, "The 'transactionManager' argument must not be null.");
this.transactionTemplate = new TransactionTemplate(transactionManager);
}
public Object someServiceMethod() {
return transactionTemplate.execute(new TransactionCallback() {
// the code in this method executes in a transactional context
public Object doInTransaction(TransactionStatus status) {
updateOperation1();
return resultOfUpdateOperation2();
}
});
}
}
If there is no return value, use the convenient
TransactionCallbackWithoutResult
class via an anonymous class:
transactionTemplate.execute(new TransactionCallbackWithoutResult() {
protected void doInTransactionWithoutResult(TransactionStatus status) {
updateOperation1();
updateOperation2();
}
});
Code within the callback can roll the transaction back by calling the
setRollbackOnly()
method on the supplied
TransactionStatus
object.
transactionTemplate.execute(new TransactionCallbackWithoutResult() {
protected void doInTransactionWithoutResult(TransactionStatus status) {
try {
updateOperation1();
updateOperation2();
} catch (SomeBusinessExeption ex) {
status.setRollbackOnly();
}
}
});
Using a
PlatformTransactionManager
You can perform transaction management using
org.springframework.transaction.PlatformTransactionManager
by completing
the following steps:-
1.
Pass the implementation of the
PlatformTransactionManager
to the bean.
84
Configuring Spring Applications on NonStop Systems