Example 5: digital in data collection, Data and string manipulation – Vernier LabPro User Manual
Page 90
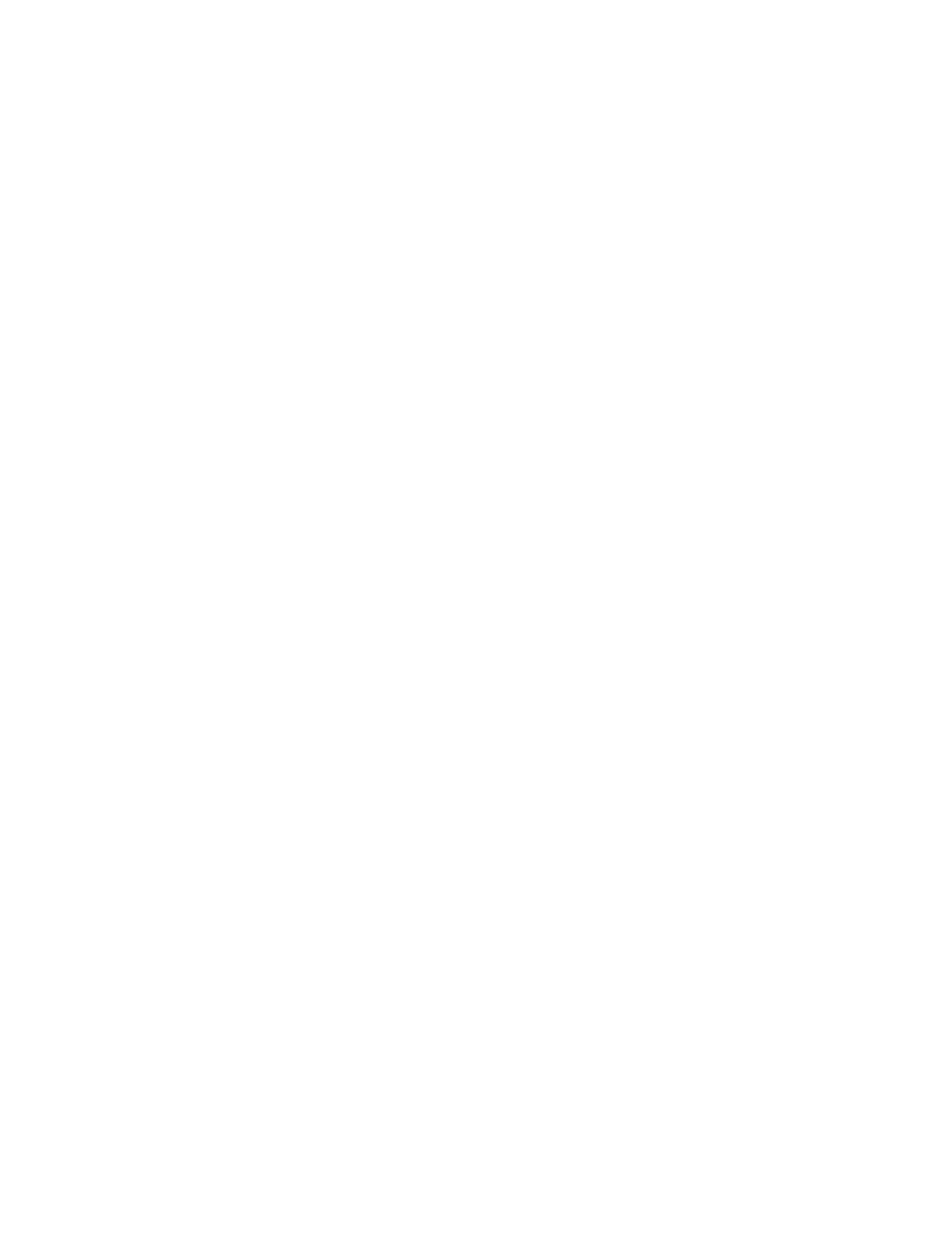
Revision Date: 08/02/02
LabPro Technical Manual
D-3
Private Sub Form_Load()
your forms load event
LabPro.PortOpen = True
opens the Comm port
LabPro.Settings = "38400,N,8,1"
sets the proper settings for LabPro
End Sub
Private Sub cmdSend_Click()
your button's click event
LabPro.Output = "s{0}" + vbCrLf
reset LabPro (clear RAM)
LabPro.Output = "s{1,31,16,0,1,2,3,4,5,6,7
sets up Channel 31 to output
the listed
,8,9,10,11,12,13,14,15}" + vbCrLf
values to digital in source
LabPro.Output = "s{3,0.5,10,0}" + vbCrLf
every 0.5 seconds send the
digital out value
End Sub
Private Sub Form_Unload(Cancel As Integer)
your forms unload event
LabPro.PortOpen = False
closes the Comm port
End Sub
Example 5: Digital In Data Collection
In this example we AutoID the probe, set up Channel 21, and send a command to collect 100 points in
metric units ( a point every 0.1 seconds). The onComm() event is called whenever data is transferred and
is used to update the listbox with new data.
Create a standard .exe file
Open the Project menu and click on Components, add a Microsoft Comm Control
Name the Comm Control LabPro
Add a command button and name it cmdCollect
Add a listbox and name it lstInput
Add the following code to your form:
Private Sub Form_Load()
your forms load event
LabPro.PortOpen = True
opens the Comm port
LabPro.Settings = "38400,N,8,1"
sets the proper settings for LabPro
End Sub
Private Sub cmdcollect_Click()
your button's click event
LabPro.Output = "s{0}" + vbCrLf
reset LabPro (clear RAM)
LabPro.Output = "s{1,21, 1}" + vbCrLf
sets up Channel 21 for digital data
collection
LabPro.Output = "s{3,0.1,100}" + vbCrLf
take sample every 0.1 second
(100 samples)
LabPro.Output = "g" + vbCrLf
"get" data command
End Sub
Private Sub labpro_OnComm()
your Comm port's communication event
lstInput.AddItem LabPro.Input
adds the data to your listbox
End Sub
Private Sub Form_Unload(Cancel As Integer)
your forms unload event
LabPro.PortOpen = False
closes the Comm port
End Sub
Data and String Manipulation
The data you receive from LabPro, in these examples, is in String format. For a real or non-real collection
a string of data would look like: { +4.40904E+00,+4.40904E+00, +4.40904E+00 }. So if you want to
access this data and receive numerical values you need to use string manipulation. The easiest way to do
this is by using the Mid function. It returns a portion of a string or caption. For example: if you want to
store the input value as a Double. You could use the following code:
(We will use labpro.Input = { +4.40904E+00 } for this example.)
If Mid(labpro.Input, 15, 1) = "0" Then returns the exponent (0)
REM input value is equal to 4.409