Appendix d: computer programming examples, General structure of a vb program, Example 2: temperature realtime data collection – Vernier LabPro User Manual
Page 88
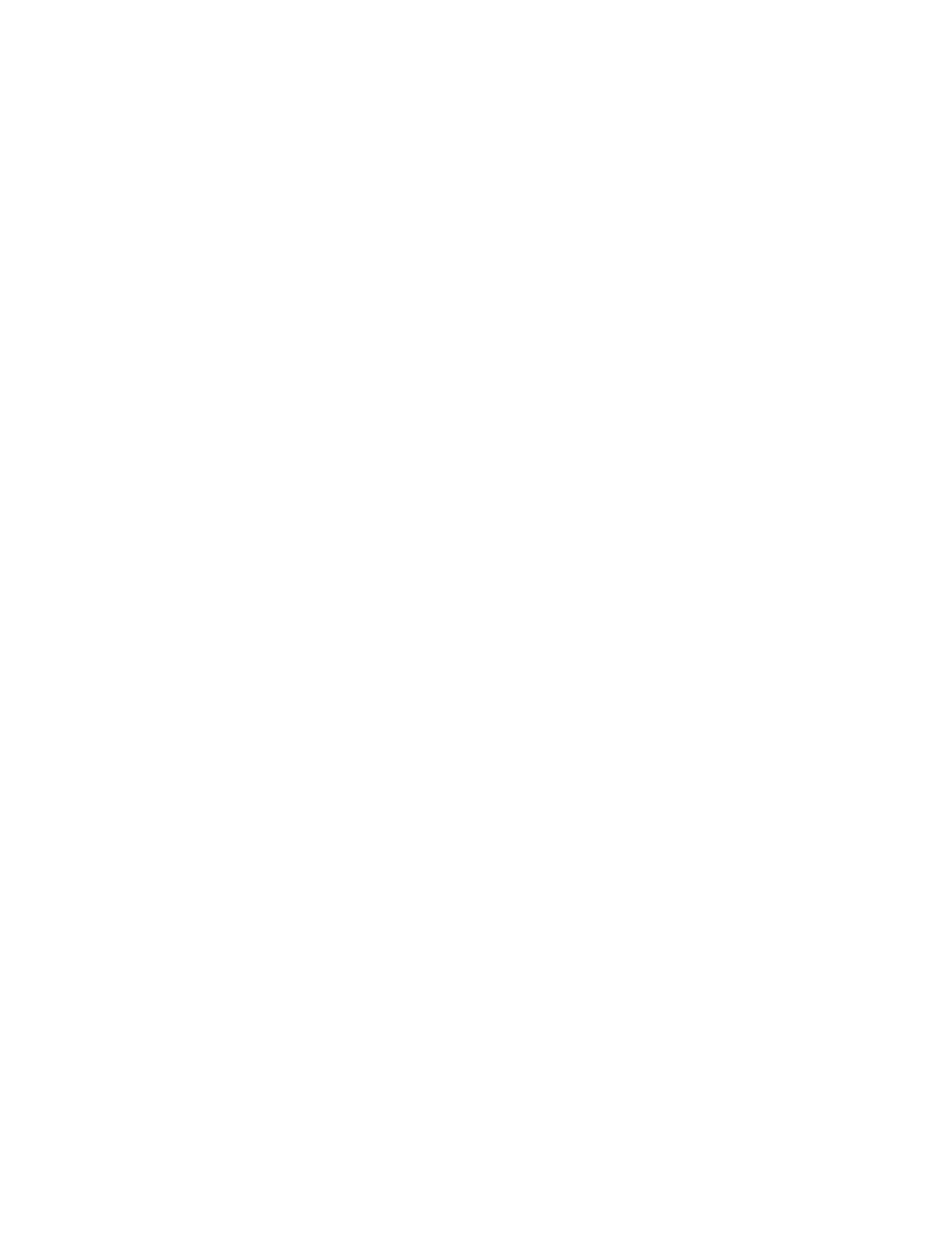
Revision Date: 08/02/02
LabPro Technical Manual
D-1
Appendix D: Computer Programming Examples
These programs were created in Microsoft Visual Basic 6.0 to set up specific LabPro operations. Samples
of various types of programs follow. Each program includes both program commands and comments
explaining what the commands do.
General Structure of a VB Program
Labpro.Output = "s{0} + vbCrLf resets LabPro (clears RAM)
Labpro.Output = "s{1,……}"
sends the initialize command to set up collection
Labpro.Output = "s{3,……}"
sends the sample rate, data points, triggering info
Channels 1-3 are Analog, Channel 21 is Digital in, Channel 11 is SONIC, and Channel 31 is Digital out.
Example 1: Temperature Non-Realtime Data Collection
In this example we AutoID the probe, set up channel 1, and send a command to collect 100 points in
metric units ( a point every 0.1 seconds). The onComm() event is called whenever data is transferred and
is used to update the listbox with new data.
Create a standard .exe file
Open the Project menu and click on Components, add a Microsoft Comm Control
Name the Comm Control LabPro
Add a command button and name it cmdCollect
Add a listbox and name it lstInput
Add the following code to your form:
Private Sub Form_Load()
your forms load event
LabPro.PortOpen = True
opens the Comm port
LabPro.Settings = "38400,N,8,1"
sets the proper settings for LabPro
End Sub
Private Sub cmdCollect_Click() your button's click event
LabPro.Output = "s{0}" + vbCrLf
reset LabPro (clears RAM)
LabPro.Output = "s{1,1,1}" + vbCrLf
set up channel 1 for collection
LabPro.Output = "s{3,.1,100,0}" + vbCrLf
takes a sample every 0.1
second (100 samples)
LabPro.Output = "g " + vbCrLf
"get" data command
End Sub
Private Sub labpro_OnComm()
your Comm port's communication event
lstInput.AddItem LabPro.Input
adds the data to your listbox
End Sub
Private Sub Form_Unload(Cancel As Integer)
your forms unload event
LabPro.PortOpen = False
closes the Comm port
End Sub
Example 2: Temperature Realtime Data Collection
In this example we AutoID the probe, set up channel 3, and send a command to collect points in realtime
in metric units. cmdStop is used to tell LabPro to stop collecting data and to return the data.
Create a standard .exe file
Open the Project menu and click on Components, add a Microsoft Comm Control
Name the Comm Control LabPro
Add a command button and name it cmdCollect
Add another command button and name it cmdStop
Add a listbox and name it lstInput
Add the following code to your form: