Reading a counter input channel – Measurement Computing DAQFlex User Guide User Manual
Page 18
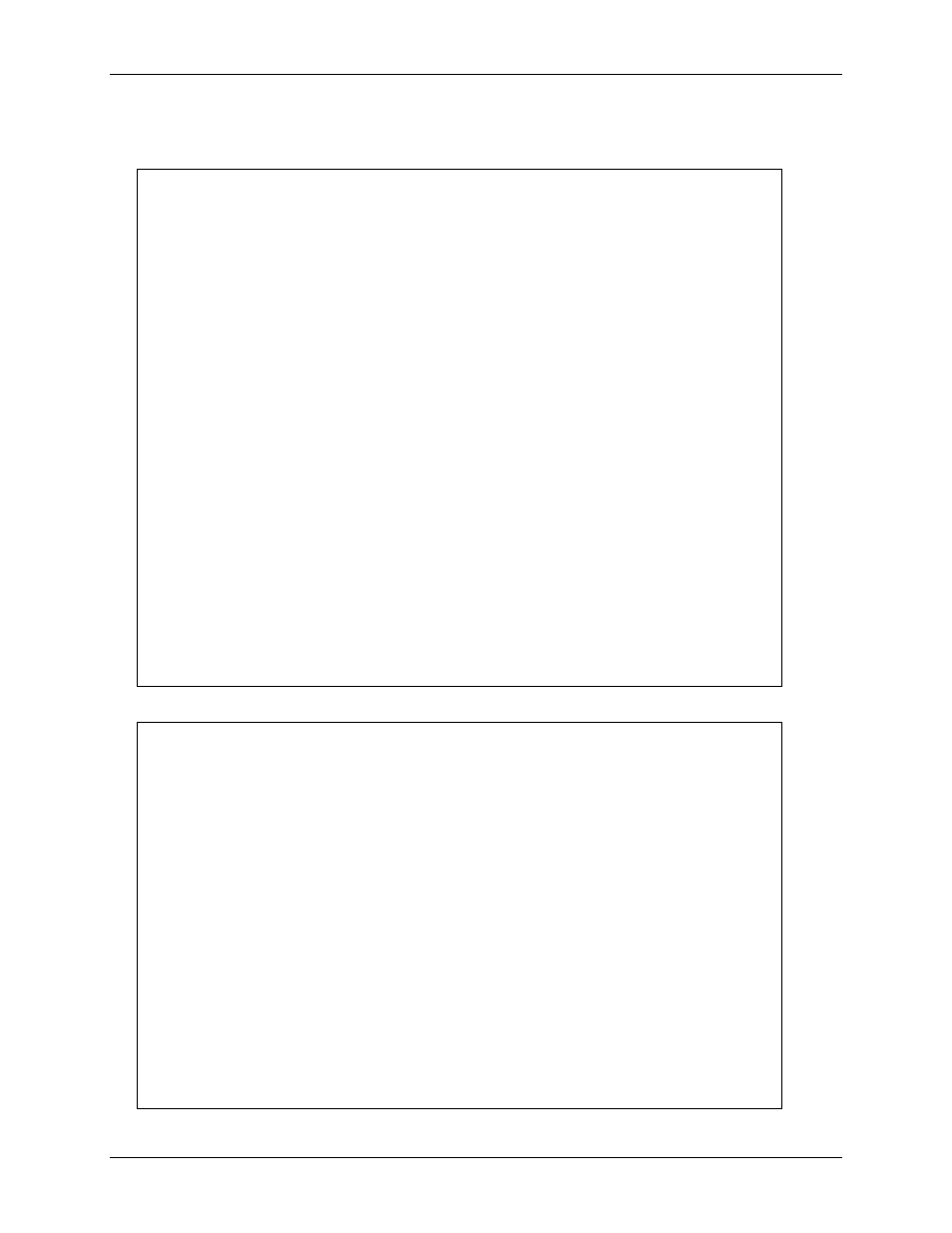
DAQFlex Software User's Guide
Using DAQFlex Software
18
Reading a counter input channel
C#
// Read counter channel 0
String[] Devices;
DaqDevice MyDevice;
DaqResponse Response;
try
{
// Get a list of message-based DAQ devices
Devices = DaqDeviceManager.GetDeviceNames(DeviceNameFormat.NameAndSerno);
// Get a DaqDevice object for device 0
MyDevice = DaqDeviceManager.CreateDevice(Devices(0));
// Start the counter
MyDevice.SendMessage("CTR{0}:VALUE=0");
MyDevice.SendMessage("CTR{0}:START");
// Read and display the daq response
for(int i = 1;i<=10;i++)
{
System.Threading.Thread.Sleep(750);
Response = MyDevice.SendMessage("?CTR{0}:VALUE");
label1.Text = Response.ToString();
Application.DoEvents();
}
// Stop the counter
MyDevice.SendMessage("CTR{0}:STOP");
}
catch (Exception ex)
{
// handle error
label1.Text = ex.Message;
}
VB
' Read counter channel 0
Dim MyDevice As DaqDevice
Dim Response As DaqResponse
Dim Devices As String ()
Try
' Get a list of message-based DAQ devices
Devices = DaqDeviceManager.GetDeviceNames(DeviceNameFormat.NameAndSerno)
' Get a DaqDevice object for device 0
MyDevice = DaqDeviceManager.CreateDevice(Devices(0))
Dim I As Integer
' Start the counter
MyDevice.SendMessage("CTR{0}:VALUE=0")
MyDevice.SendMessage("CTR{0}:START")
' Read and display the daq response
For I = 1 To 10
System.Threading.Thread.Sleep(750)
Response = MyDevice.SendMessage("?CTR{0}:VALUE")
Label1.Text = Response.ToString()
Application.DoEvents()
Next