Control structures – Pololu Maestro User Manual
Page 51
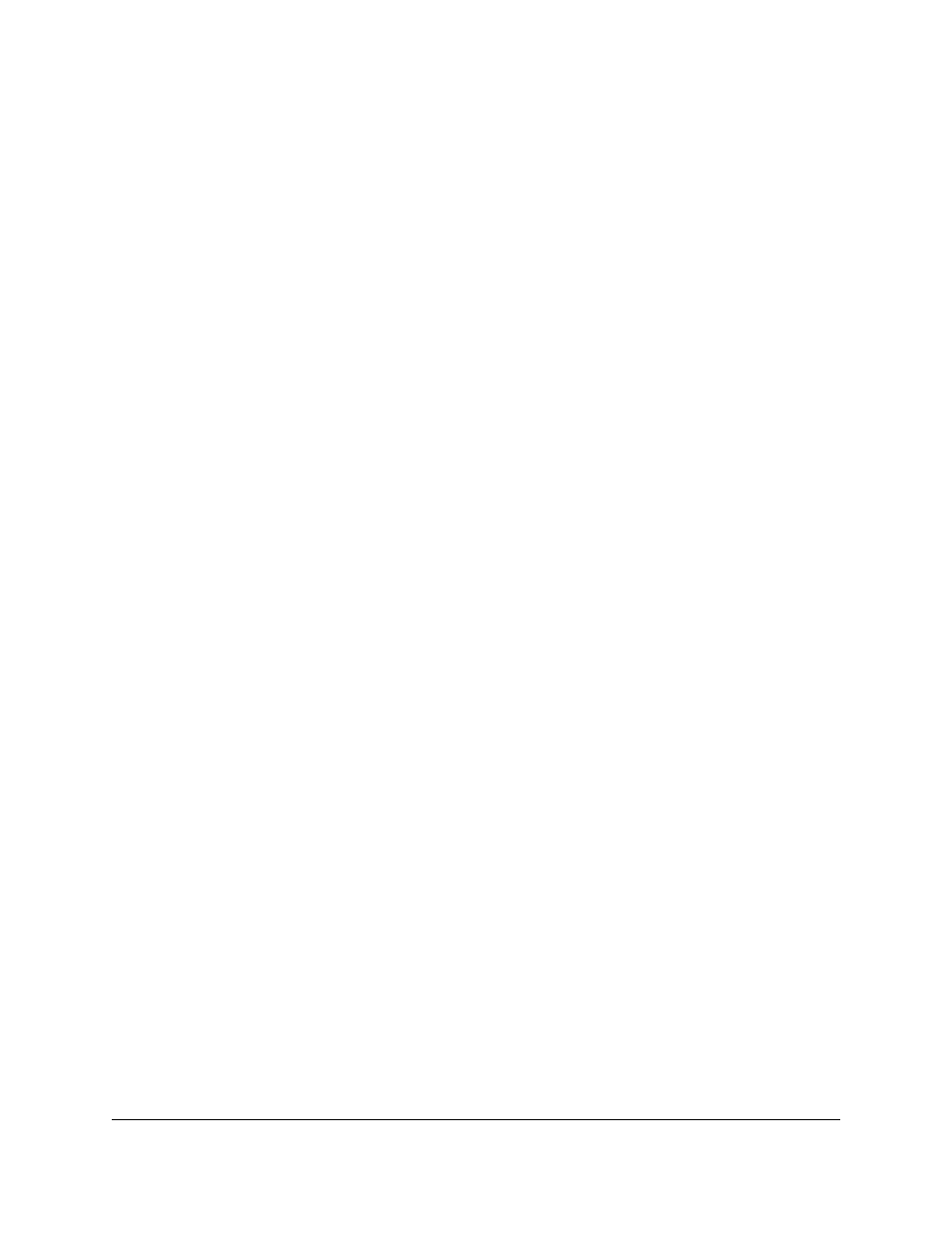
1 3 miNUS
4 # this is a comment!
times
with absolutely no effect on the compiled program. We generally use lower-case for commands and two or four spaces
of indentation to indicate control structures and subroutines, but feel free to arrange your code to suit your personal
style.
Control structures
The Maestro script language has several control structures, which allow arbitrarily complicated programs to be
written. Unlike subroutines, there is no limit to the level of nesting of control structures, since they are all ultimately
based on GOTO commands (discussed below) and simple branching. By far the most useful control structure is the
BEGIN…REPEAT infinite loop, an example of which is given below:
# move servo 1 back and forth with a period of 1 second
begin
8000 1 servo
500 delay
4000 1 servo
500 delay
repeat
This infinite loop will continue forever. If you want a loop that is bounded in some way, the WHILE keyword is likely
to be useful. WHILE consumes the top number on the stack and jumps to the end of the loop if and only if it is a zero.
For example, suppose we want to repeat this loop exactly 10 times:
10 # start with a 10 on the stack
begin
dup # copy the number on the stack - the copy will be consumed by WHILE
while # jump to the end if the count reaches 0
8000 1 servo
500 delay
4000 1 servo
500 delay
1 minus # subtract 1 from the number of times remaining
repeat
Note that BEGIN…WHILE…REPEAT loops are similar to the while loops of many other languages. But, just like
everything else in the Maestro script language, the WHILE comes after its argument.
For conditional actions, an IF…ELSE…ENDIF structure is useful. Suppose we are building a system with a sensor
on channel 3, and we want to set servo 5 to 6000 (1.5 ms) if the input value is less than 512 (about 2.5 V). Otherwise,
we will set the servo to 7000 (1.75 ms). The following code accomplishes this relatively complicated task:
3 get_position # get the value of input 3 as a number from 0 to 1023
512 less_than # test whether it is less than 512 -> 1 if true, 0 if false
if
6000 5 servo # this part is run when input3 < 512
else
7000 5 servo # this part is run when input3 >= 512
endif
As in most languages, the ELSE section is optional. Note again that this seems at first to be backwards relative to
other languages, since the IF comes after the test.
The WHILE and IF structures are enough to create just about any kind of script. However, there are times when it is
just not convenient to think about what you are trying to do in terms of a loop or a branch. If you just want to jump
directly from one part of code to another, you may use a GOTO. It works like this:
Pololu Maestro Servo Controller User's Guide
© 2001–2014 Pololu Corporation
6. The Maestro Scripting Language
Page 51 of 73