Using the fml buffer type – HP NonStop G-Series User Manual
Page 75
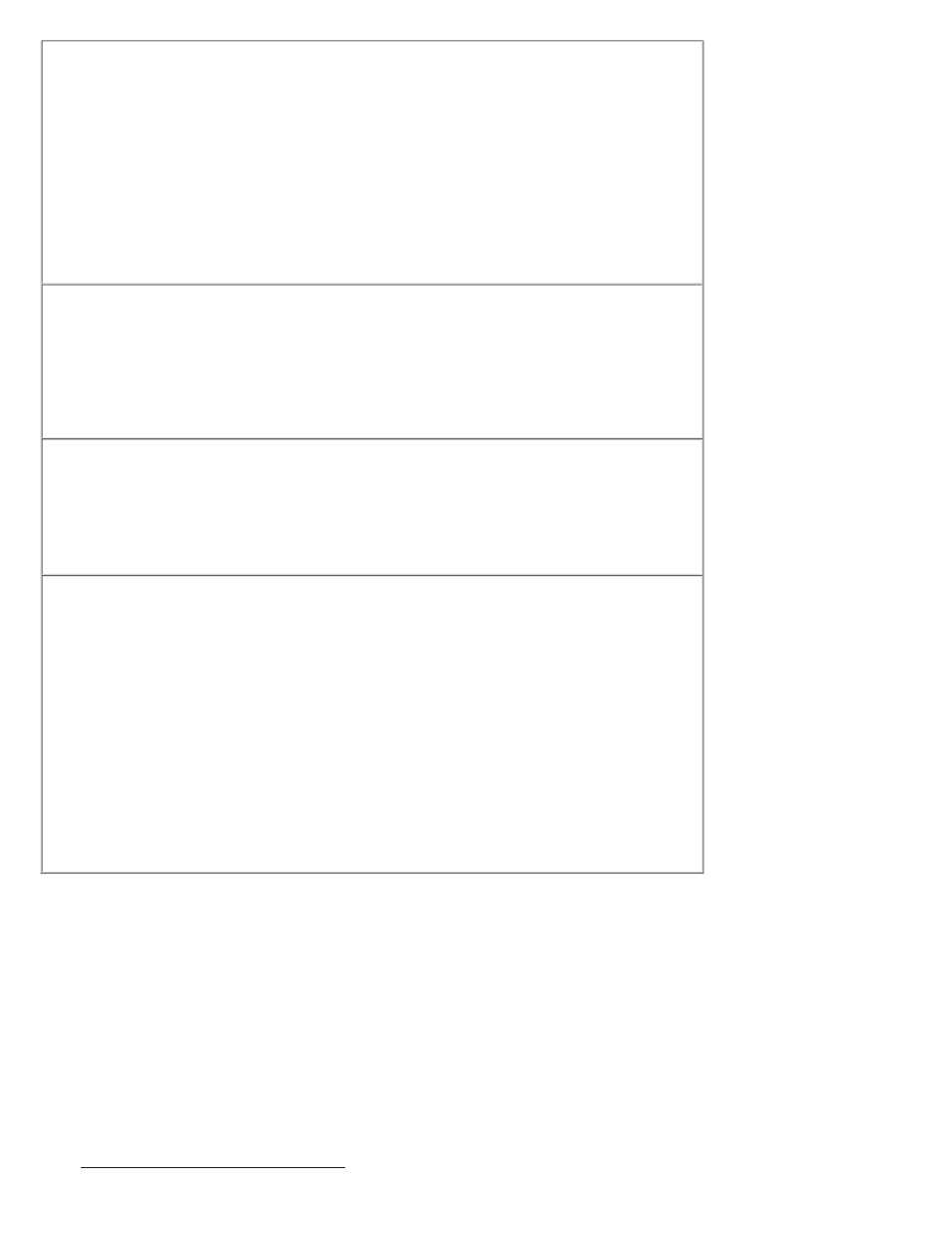
import java.io.*;
import bea.jolt.*;
class ...
{
...
public void tryOnCARRAY()
{
byte data[];
JoltRemoteService csvc;
DataInputStream din;
DataOutputStream dout;
ByteArrayInputStream bin;
ByteArrayOutputStream bout;
/* Use java.io.DataOutputStream to put data into a byte array
*/
bout = new ByteArrayOutputStream(512);
dout = new DataOutputStream(bout);
dout.writeInt(100);
dout.writeFloat((float) 300.00);
dout.writeUTF("Hello World");
dout.writeShort((short) 88);
/* Copy the byte array into a new byte array "data". Then
* issue the Jolt remote service call.
*/
data = bout.toByteArray();
csvc = new JoltRemoteService("ECHO", session);
csvc.setBytes("CARRAY", data, data.length);
csvc.call(null);
/* Get the result from JoltRemoteService object and use
* java.io.DataInputStream to extract each individual value
* from the byte array.
*/
data = csvc.getBytesDef("CARRAY", null);
if (data != null)
{
bin = new ByteArrayInputStream(data);
din = new DataInputStream(bin);
System.out.println(din.readInt());
System.out.println(din.readFloat());
System.out.println(din.readUTF());
System.out.println(din.readShort());
}
}
}
Using the FML Buffer Type
FML (Field Manipulation Language) is a flexible data structure that can be used as a typed buffer. The FML data structure stores tagged
values that are typed, variable in length, and may have multiple occurrences. The typed buffer is treated as an abstract data type in FML.
FML gives you the ability to access and update data values without having to know how the data is structured and stored. In your
application program, you simply access or update a field in the fielded buffer by referencing its identifier. To perform the operation, the
FML runtime determines the field location and data type.
FML is especially suited for use with Jolt clients as the client and server code may be in two languages (e.g., Java and C), the client/server
platforms may have different data type specifications, or the interface between the client and the server changes frequently.
The following tryOnFml examples illustrate the use of the FML typed buffer. The examples show a Jolt client using FML buffers to pass
data to a server. The server takes the buffer, creates a new FML buffer to store the data, and passes that buffer back to the Jolt client. The
examples consist of the following components.
Example 6-4, tryOnFml.java Source Code
is the Jolt client that contains a PASSFML service.
●