Working with sql/mx, Integration with nsmq, Working with sql/mx integration with nsmq – HP Integrity NonStop H-Series User Manual
Page 92
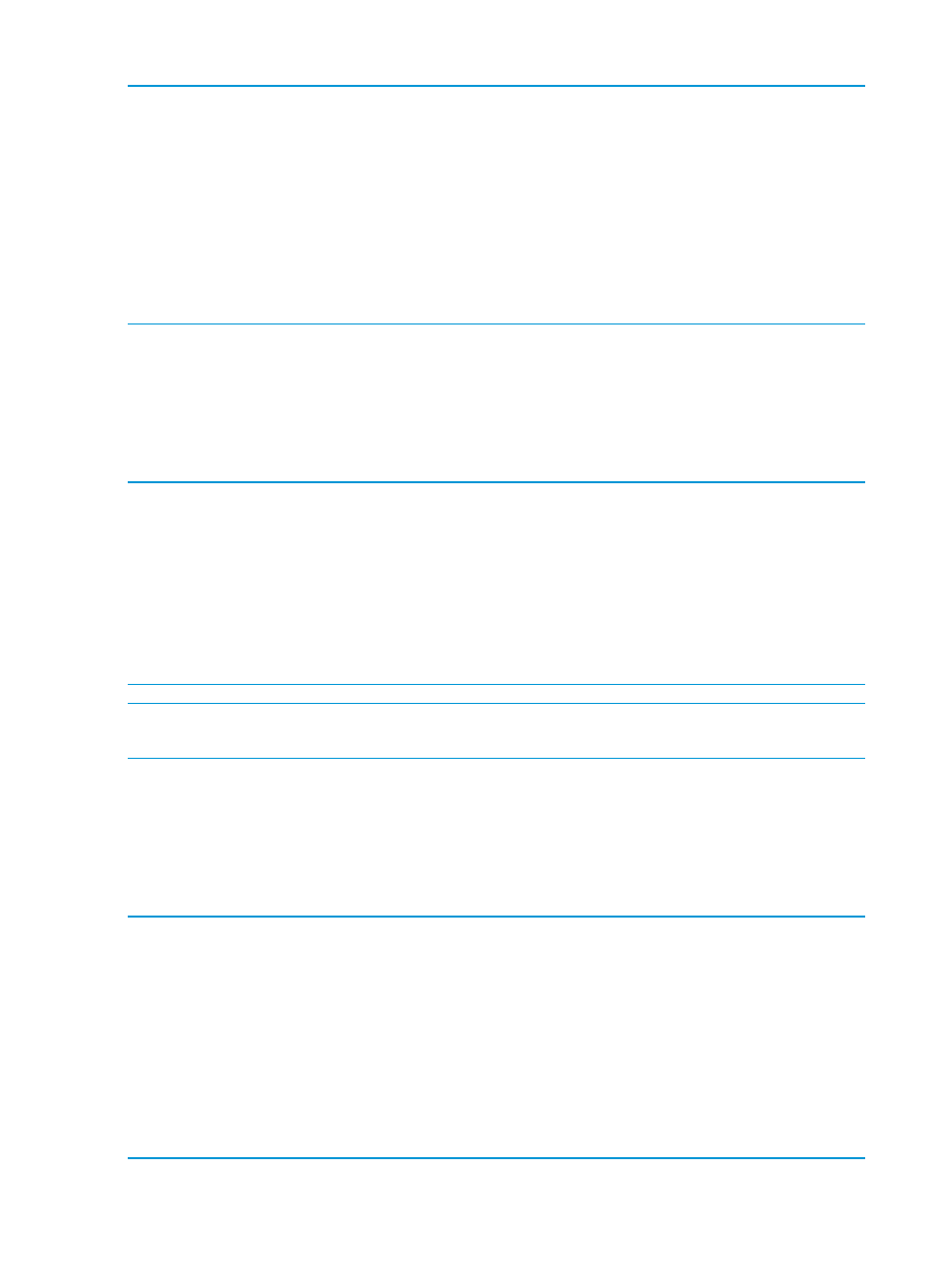
Example 3 Sample snippet
public class SomeServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@EJB(mappedName = "java:global/SomeSessionBean/SomeBean!com.hp.nsasj.sample.SomeBean")
SomeBean bean;
public SomeServlet() {
super();
}
protected void doGet(HttpServletRequest request,
HttpServletResponse response)
throws ServletException, IOException {
PrintWriter out = response.getWriter();
out.println(bean.sayHello());
}
}
Working with SQL/MX
Using datasource configuration, and with the help of JDBC T2 or T4 driver, the Servlets can persist
data to the SQL/MX database. The configuration is similar to NSASJ for EJB. Hibernate packaged
with NSASJ and the hibernate dialect for SQL/MX can be used for persistence using JPA.
Example 4 Sample snippet
Assuming a datasource called Test is declared, the following snippet needs to be added in the
Servlet’s init method:
DataSource ds = null;
Context ctx = null;
try {
String strDSName = "java:jboss/datasources/Test";
ctx = new InitialContext();
ds = (javax.sql.DataSource) ctx.lookup(strDSName);
} catch (Exception e) {
}
NOTE:
Depending on the driver specified in the Test Datasources configuration and the datasource
attributes, the appropriate driver is used for connecting to the database.
Integration with NSMQ
NSASJ is integrated with NSMQ through the resource archive file. Servlets can act as message
producers and can send messages to a queue or topic. This feature is tested with a sample. The
simplest way is to inject JMS as resources.
Example 5 Sample snippet
public class SomeServlet extends HttpServlet {
@Resource(mappedName = "queue/testQueue")
private Queue queueExample;
@Resource(mappedName = "java:/ConnectionFactory")
private ConnectionFactory cf;
public void sendMessage() {
connection = cf.createConnection();
. . . .
}
}
92
Integration