Moog Crossbow GNAV540 User Manual
Page 118
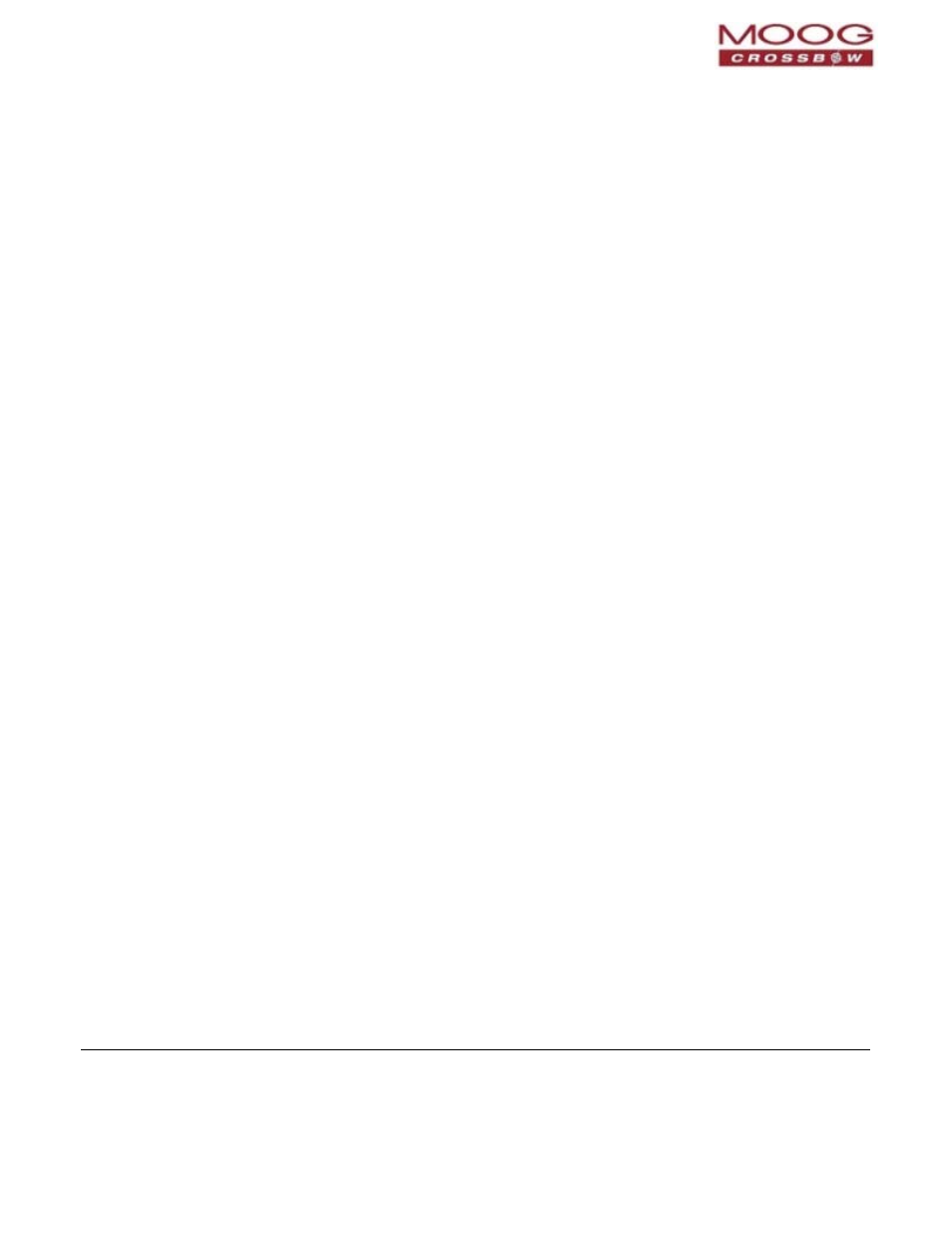
Page 118
GNAV540 User Manual
7430‐0808‐01 Rev. B
/*******************************************************************************
* FUNCTION: DeleteQeue - return an item from the queue
* ARGUMENTS: item will hold item popped from queue
*
queue_ptr is pointer to the queue
* RETURNS:
returns 0 if queue is empty. 1 if successful
*******************************************************************************/
int DeleteQueue(char *item, QUEUE_TYPE *queue_ptr)
{
int retval = 0;
if(queue_ptr->count <= 0)
{
retval = 0; /* queue is empty */
}
else
{
queue_ptr
->
count--;
*item
=
queue_ptr->entry(queue_ptr->front);
queue_ptr->front = (queue_ptr->front+1) % MAXQUEUE;
retval=1;
}
return
retval;
}
/*******************************************************************************
* FUNCTION: peekByte returns 1 byte from buffer without popping
* ARGUMENTS: queue_ptr is pointer to the queue to return byte from
*
index is offset into buffer to which byte to return
* RETURNS:
1 byte
* REMARKS:
does not do boundary checking. please do this first
*******************************************************************************/
char peekByte(QUEUE_TYPE *queue_ptr, unsigned int index) {
char
byte;
int
firstIndex;
firstIndex = (queue_ptr->front + index) % MAXQUEUE;
byte = queue_ptr->entry(firstIndex);
return byte;
}
/*******************************************************************************
* FUNCTION: peekWord returns 2-byte word from buffer without popping
* ARGUMENTS: queue_ptr is pointer to the queue to return word from
*
index is offset into buffer to which word to return
* RETURNS:
2-byte word
* REMARKS:
does not do boundary checking. please do this first
*******************************************************************************/
unsigned short peekWord(QUEUE_TYPE *queue_ptr, unsigned int index) {
unsigned short word, firstIndex, secondIndex;
firstIndex = (queue_ptr->front + index) % MAXQUEUE;