Pololu Simple User Manual
Page 94
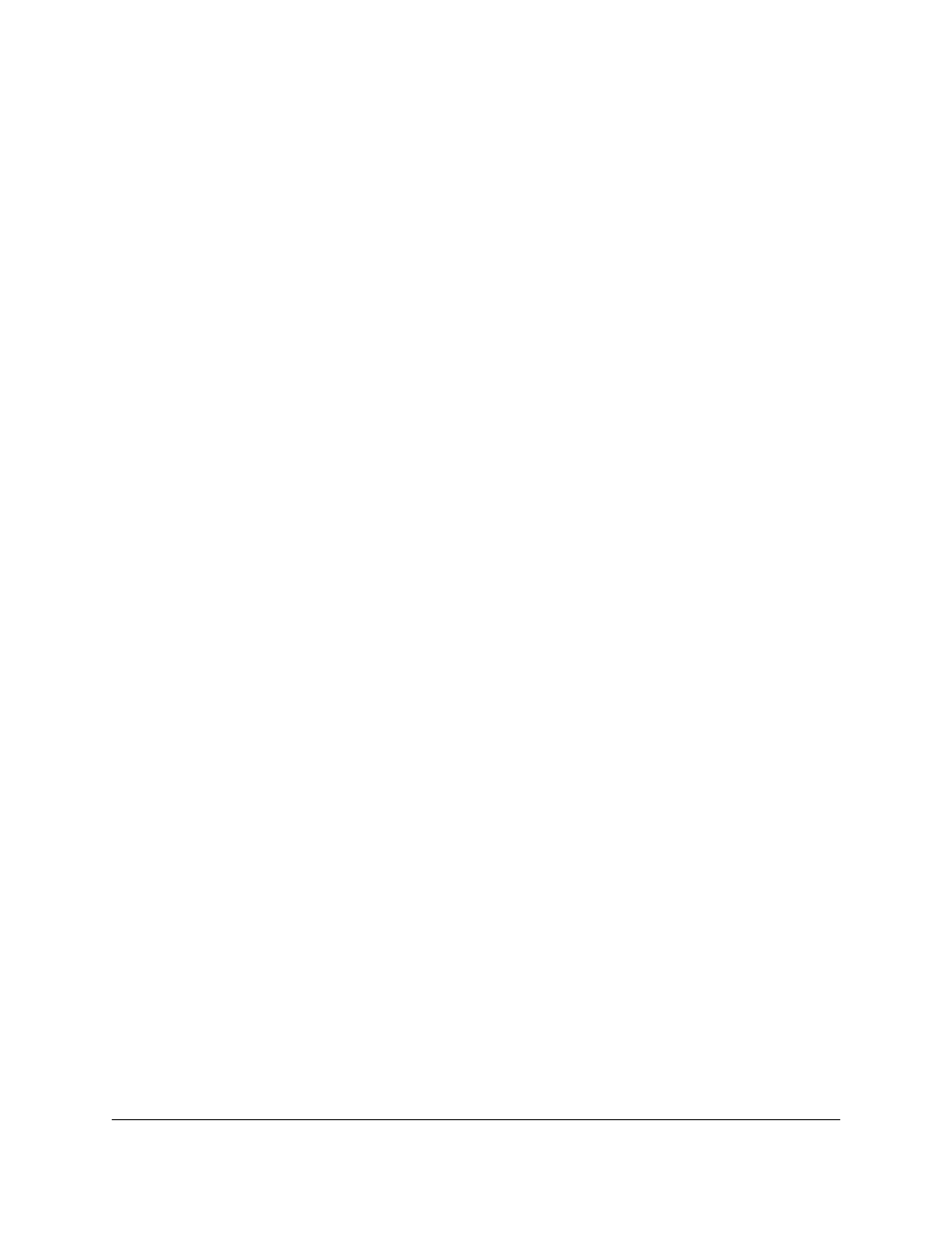
char command[4];
// These first three functions call the appropriate Pololu AVR library serial functions
// depending on which Orangutan you are using. The Orangutan SVP and X2 have multiple
// serial ports, so the serial functions for these devices require an extra argument
// specifying which port to use. You can simplify this program by just calling the
// library function appropriate for your Orangutan board.
void setBaudRate(unsigned long baud)
{
#if _SERIAL_PORTS > 1 // Orangutan X2 and SVP users
serial_set_baud_rate(UART0, baud);
#else
serial_set_baud_rate(baud);
#endif
}
void sendBlocking(char * buffer, unsigned char size)
{
#if _SERIAL_PORTS > 1 // Orangutan X2 and SVP users
serial_send_blocking(UART0, buffer, size);
#else
serial_send_blocking(buffer, size);
#endif
}
char receiveBlocking(char * buffer, unsigned char size, unsigned int timeout_ms)
{
#if _SERIAL_PORTS > 1 // Orangutan X2 and SVP users
return serial_receive_blocking(UART0, buffer, size, timeout_ms);
#else
return serial_receive_blocking(buffer, size, timeout_ms);
#endif
}
// required to allow motors to move
// must be called when controller restarts and after any error
void exitSafeStart()
{
command[0] = 0x83;
sendBlocking(command, 1);
}
// speed should be a number from -3200 to 3200
void setMotorSpeed(int speed)
{
if (speed < 0)
{
command[0] = 0x86; // motor reverse command
speed = -speed; // make speed positive
}
else
{
command[0] = 0x85; // motor forward command
}
command[1] = speed & 0x1F;
command[2] = speed >> 5;
sendBlocking(command, 3);
}
char setMotorLimit(unsigned char limitID, unsigned int limitValue)
{
command[0] = 0xA2;
command[1] = limitID;
command[2] = limitValue & 0x7F;
command[3] = limitValue >> 7;
sendBlocking(command, 4);
char response = -1;
receiveBlocking(&response, 1, 500);
return response;
}
// returns the specified variable as an unsigned integer.
// if the requested variable is signed, the value returned by this function
// should be typecast as an int.
unsigned int getVariable(unsigned char variableID)
Pololu Simple Motor Controller User's Guide
© 2001–2014 Pololu Corporation
6. Using the Serial Interface
Page 94 of 101