Pololu Simple User Manual
Page 90
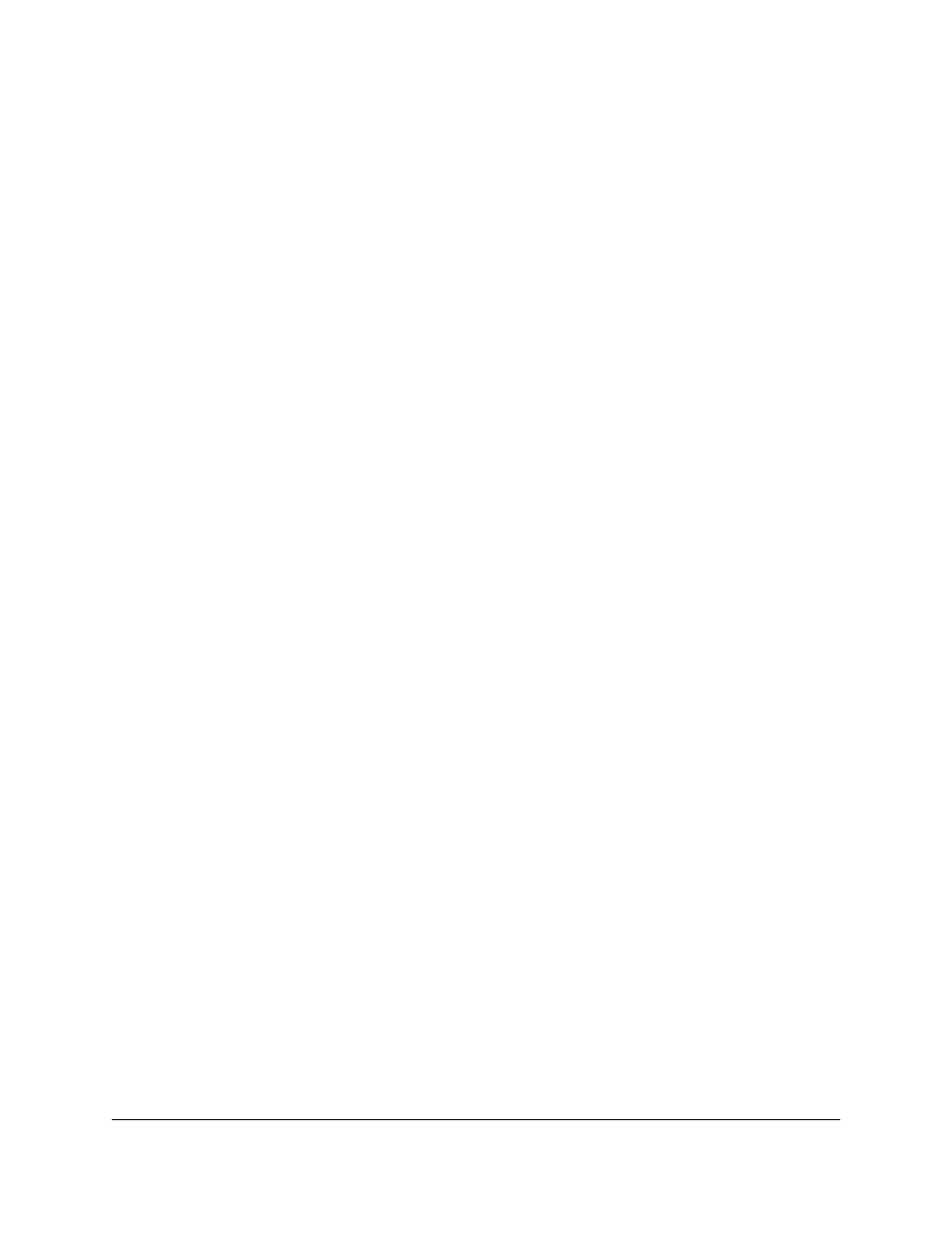
#define ERROR_STATUS 0
#define LIMIT_STATUS 3
#define TARGET_SPEED 20
#define INPUT_VOLTAGE 23
#define TEMPERATURE 24
// some motor limit IDs
#define FORWARD_ACCELERATION 5
#define REVERSE_ACCELERATION 9
#define DECELERATION 2
// read a serial byte (returns -1 if nothing received after the timeout expires)
int readByte()
{
char c;
if(smcSerial.readBytes(&c, 1) == 0){ return -1; }
return (byte)c;
}
// required to allow motors to move
// must be called when controller restarts and after any error
void exitSafeStart()
{
smcSerial.write(0x83);
}
// speed should be a number from -3200 to 3200
void setMotorSpeed(int speed)
{
if (speed < 0)
{
smcSerial.write(0x86); // motor reverse command
speed = -speed; // make speed positive
}
else
{
smcSerial.write(0x85); // motor forward command
}
smcSerial.write(speed & 0x1F);
smcSerial.write(speed >> 5);
}
unsigned char setMotorLimit(unsigned char limitID, unsigned int limitValue)
{
smcSerial.write(0xA2);
smcSerial.write(limitID);
smcSerial.write(limitValue & 0x7F);
smcSerial.write(limitValue >> 7);
return readByte();
}
// returns the specified variable as an unsigned integer.
// if the requested variable is signed, the value returned by this function
// should be typecast as an int.
unsigned int getVariable(unsigned char variableID)
{
smcSerial.write(0xA1);
smcSerial.write(variableID);
return readByte() + 256 * readByte();
}
void setup()
{
Serial.begin(115200); // for debugging (optional)
smcSerial.begin(19200);
// briefly reset SMC when Arduino starts up (optional)
pinMode(resetPin, OUTPUT);
digitalWrite(resetPin, LOW); // reset SMC
delay(1); // wait 1 ms
pinMode(resetPin, INPUT); // let SMC run again
// must wait at least 1 ms after reset before transmitting
delay(5);
// this lets us read the state of the SMC ERR pin (optional)
pinMode(errPin, INPUT);
Pololu Simple Motor Controller User's Guide
© 2001–2014 Pololu Corporation
6. Using the Serial Interface
Page 90 of 101