Data types, Typedef struct jscontext jscontext – Adobe Extending Flash Professional CS4 User Manual
Page 549
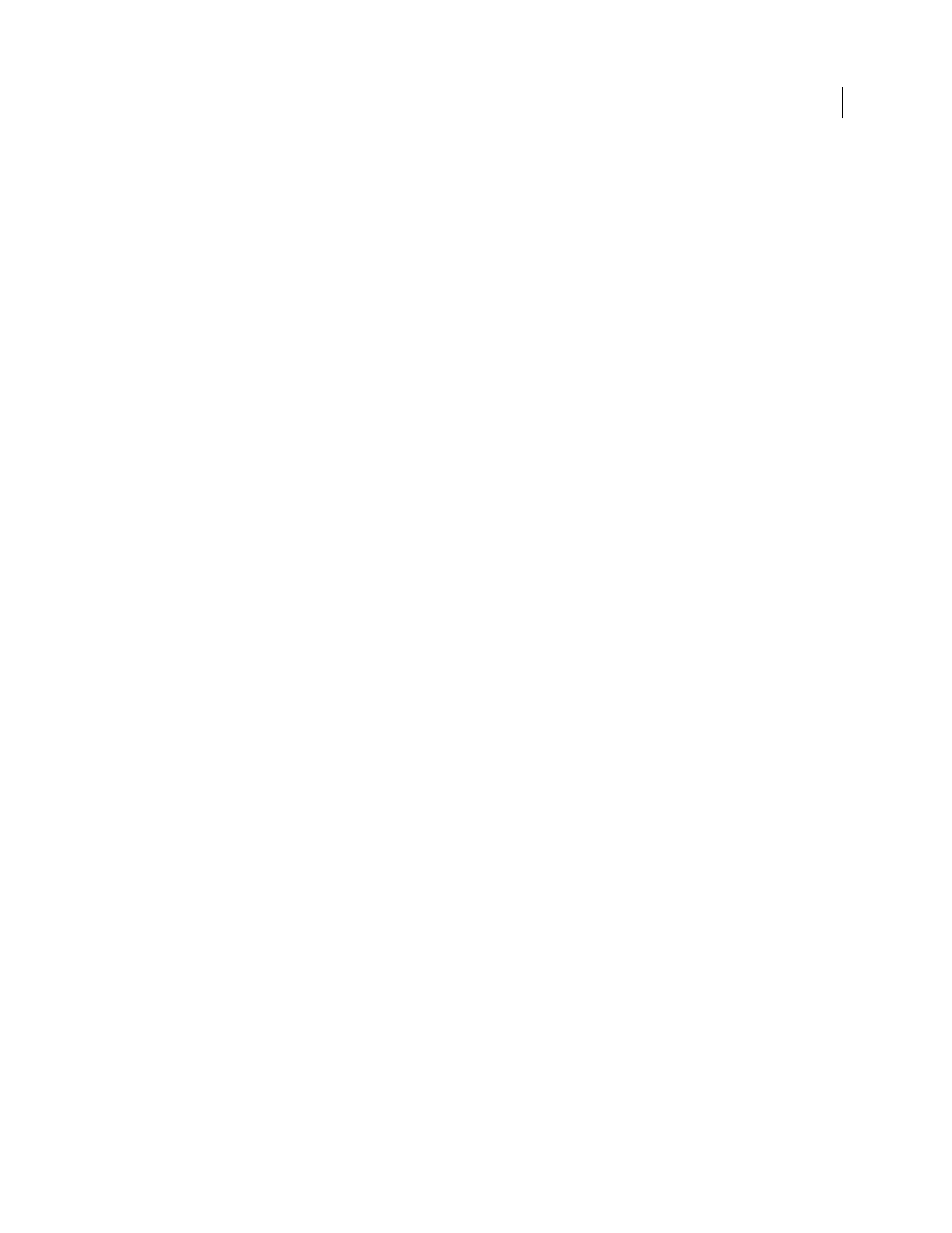
527
EXTENDING FLASH CS4 PROFESSIONAL
C-Level Extensibility
// Source code in C
// Save the DLL or shared library with the name "Sample".
#include
#include
#include "mm_jsapi.h"
// A sample function
// Every implementation of a JavaScript function must have this signature.
JSBool computeSum(JSContext *cx, JSObject *obj, unsigned int argc, jsval *argv, jsval *rval)
{
long a, b, sum;
// Make sure the right number of arguments were passed in.
if (argc != 2)
return JS_FALSE;
// Convert the two arguments from jsvals to longs.
if (JS_ValueToInteger(cx, argv[0], &a) == JS_FALSE ||
JS_ValueToInteger(cx, argv[1], &b) == JS_FALSE)
return JS_FALSE;
/* Perform the actual work. */
sum = a + b;
/* Package the return value as a jsval. */
*rval = JS_IntegerToValue(sum);
/* Indicate success. */
return JS_TRUE;
}
After writing this code, build the DLL file or shared library, and store it in the appropriate Configuration/External
Libraries directory (see “
” on page 522). Then create a JSFL file with the following code, and
store it in the Configuration/Commands directory (see “
// JSFL file to run C function defined above.
var a = 5;
var b = 10;
var sum = Sample.computeSum(a, b);
fl.trace("The sum of " + a + " and " + b + " is " + sum );
To run the function defined in the DLL, select Commands > Sample in the Flash authoring environment.
Data types
The JavaScript interpreter defines the data types described in this section.
typedef struct JSContext JSContext
A pointer to this opaque data type passes to the C-level function. Some functions in the API accept this pointer as one
of their arguments.