Adding guests to the guest list, Adding guests to the guest list 50 – Apple WebObjects 3.5 User Manual
Page 50
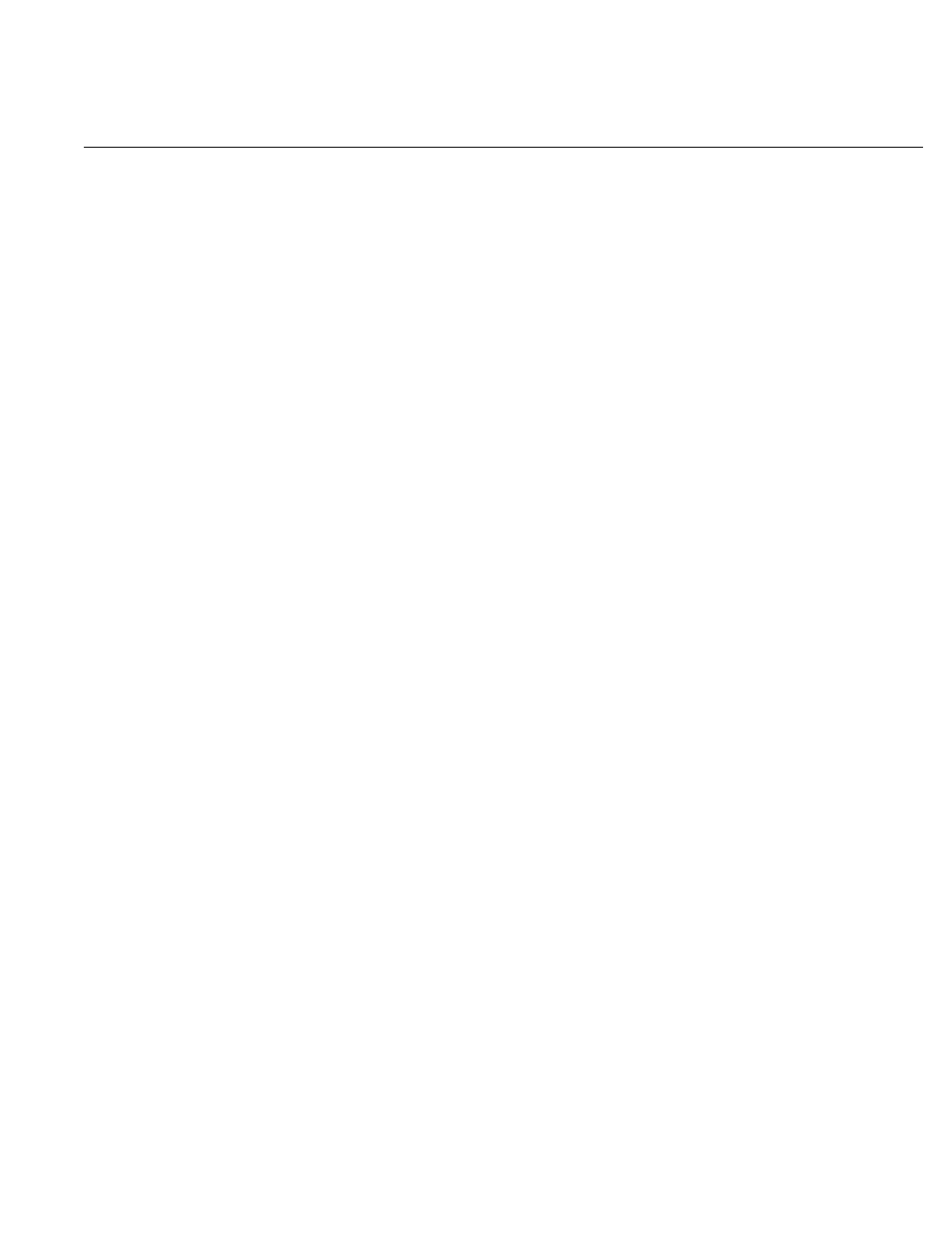
Chapter 2
Enhancing Your Application
50
3. At the top of the Application class definition, enter this declaration:
protected MutableVector allGuests;
This declares
allGuests
to be of type MutableVector. Declaring it
protected
means that it is accessible only from this class or one of its
subclasses. It is standard object-oriented practice for a class to prevent
other classes from directly manipulating its instance variables. Instead,
you provide accessor methods that other objects use to read or modify the
instance variables.
4. Add the accessor methods
addGuest
and
clearGuests
, as shown in the figure.
The
addGuest
method adds an object of class Guest to the end of the
allGuests
array, using the MutableVector method
addElement
(its Objective-C
equivalent is
addObject
).
The
clearGuests
method removes all the objects from the array using the
MutableVector method
removeAllElements
(its Objective-C equivalent is
removeAllObjects
).
5. Save
Application.java
.
Adding Guests to the Guest List
Now, when the user submits the form, you’ll add the information to the
allGuests
array rather than displaying it directly.
1. Switch to the code for
Main.java
.
2. In the
submit
method, add the following code before the
return
statement:
((Application)application()).addGuest(currentGuest);
currentGuest = new Guest();
This code calls the application’s
addGuest
method, which adds an object (in
this case,
currentGuest
) to the end of the array. Then it creates a new Guest
object to hold the next guest’s data.
Note:
The
addGuest
method is defined in the class Application, which is a
subclass of WebApplication. The component’s
application
method (called in
the above statement) returns an object of type WebApplication, so you
must cast it to Application in order to access its
addGuest
method.
Your next step is to create a new component to display the list of guests that
allGuests
stores.