Comtrol API (6508) for the MS-DOS User Manual
Page 17
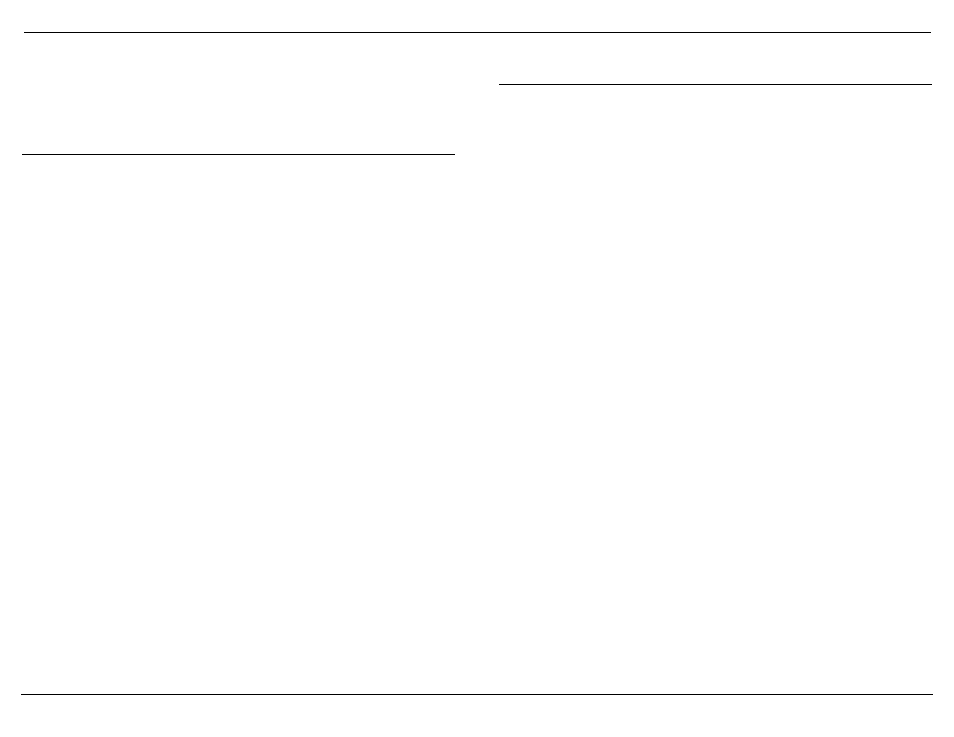
Developing Applications
17
Developing Applications
during event functions and any functions called by event functions.
Stack checking can be turned off and on with:
#pragma check_stack(off)
#pragma check_stack(on)
2.13. Double Buffering Transmit and Receive Data
Each serial device on the RocketPort controller internally provides 250 bytes
of buffering for transmit data and 1K bytes of buffering for receive data. In
some applications this may not be sufficient.
For example, an application program may need to write large blocks of data at
infrequent intervals. If the application calls aaWrite() directly, only 250 bytes
are taken, and the device's internal transmit buffer may empty before the next
aaWrite() call occurs, leaving a period of time where no data is being
transmitted.
In cases like the one described above, additional buffering is needed. To
accomplish this, the data can be double buffered using event functions (see
Subsection 2.12). This allows the application to move serial data to and from
the buffers rather than directly accessing the device using aaWrite() and
aaRead(). The event function handles moving data between the device and the
buffer. Double buffering as described in this subsection adds additional
overhead, so it should only be done when an application requires it.
A sample program (
\ROCKET\SAMPLE\DBUF.C
) shows an example of double
buffering. Also included is a Borland C++ make file called
MAKEDBUF.BC
. The
source code is reproduced in this guide in Appendix B .
For double buffering of transmit data, use the periodic event function. This
function polls each device's buffer for data, and if data is available writes it to
the device using aaWrite(). The EvPeriodic() function in
DBUF.C
shows how to do
this.
The EnqTxData() is used in
DBUF.C
to write data into the transmit buffer. The
application calls EnqTxData() instead of writing directly to the device with
aaWrite(). Notice that EnqTxData() disables interrupts while manipulating the
write buffer pointers. This is necessary because EvPeriodic() is part of an
interrupt service routine (
ISR
) and you do not want it to suddenly interrupt and
change these pointers until you are completely done updating them.
For double buffering of receive data, the receive event function should be used.
This event function is not called unless the device has receive data available.
The event function then reads the data with aaRead() or aaReadWithStatus()
and places it in the receive buffer. A simple example using only aaRead() is
shown in the EvRxData() function in
DBUF.C
.
The DeqRxData() function is used in
DBUF.C
to read data from the receive
buffer. The application calls DeqRxData() instead of reading directly from the
device with aaRead(). Notice that DeqRxData() disables interrupts while
manipulating the read buffer pointers. EvRxData() is part of an
ISR
, so this is
necessary for the same reason interrupts were disabled in EnqTxData().
2.14. Building Applications (Step 6)
The application is built by executing the compiler’s make utility and a make
file. The make file contains the rules that the make utility uses to build the
application. If the application is contained entirely in a single source file
called
TERM.C
, then the make file copied in from the
\ROCKET\SAMPLE
directory can be used as is. Otherwise, you must modify the make file to build
using your application source file names.