Comtrol API (6508) for the MS-DOS User Manual
Page 16
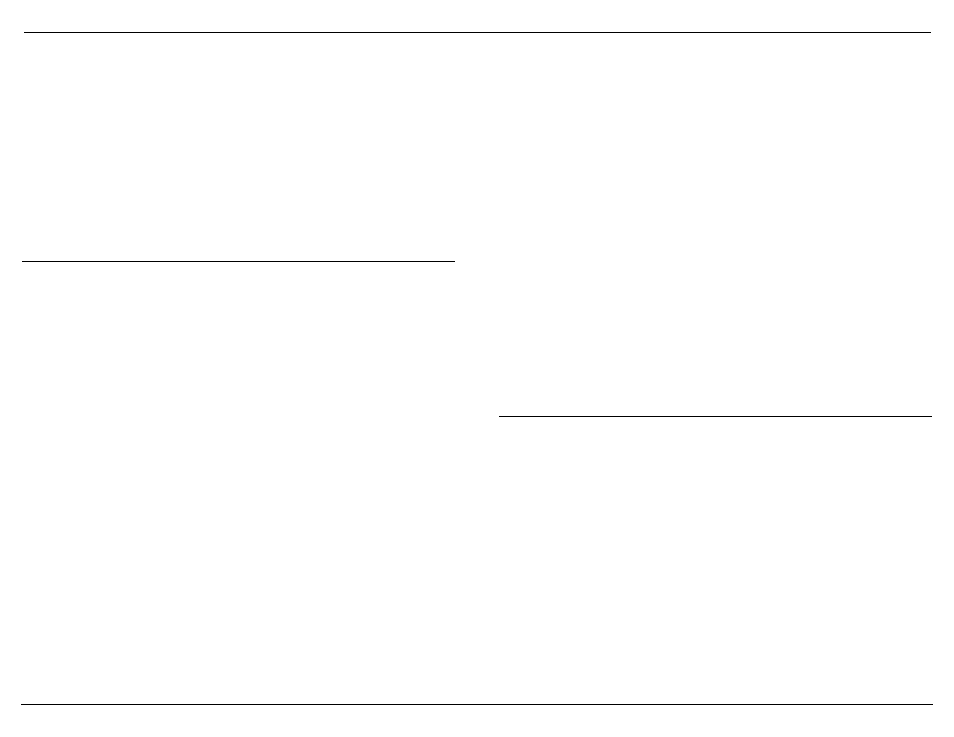
16
Developing Applications
Developing Applications
you can give the system software the name of an application program function
that executes when a particular event occurs. The following aaInstallxxxEvent
functions are available:
•
aaInstallMdmChangeEvent
•
aaInstallPeriodicEvent
•
aaInstallRxEvent
Example 2-1 provides event function examples and shows how to install event
functions. Notice that installing event functions is done shortly after the
controller is initialized.
Even after an event function is installed, it will not be dispatched unless that
event has been enabled. Modem change and receive data events are enabled or
disabled using the DetectEn parameter in the aaOpen() function. Periodic
events are enabled or disabled using the aaEnPeriodicEvent() function.
Example 2-1. Sample Event Function
#define NUMDEV 32 /* max number devices this app supports */
int FirstDev, MaxDev; /* first and maximum device numbers */
unsigned char
CD
_Change[
NUMDEV
]; /* indicates changes to
CD
modem input */
unsigned char ModemState[
NUMDEV
]; /* state of modem inputs for each device */
main() /* application main() fragment */
{ int NumDev;
/* Initialize controller */
aaInstallCtrlCHandler();
if(aaInit() !=
NO_ERR
)
{
printf("Initialization Failure\n");
aaExit();
exit(1);
}
/* Get device number range for 1 controller */
if(aaGetCtlStatus(0,&FirstDev,&NumDev) !=
NO_ERR
)
{
printf("Controller Status Failure\n");
aaExit();
exit(1);
} }
MaxDev = FirstDev + NumDev - 1;
/* Set up application event functions */
aaInstallRxEvent(EvRxData);
aaInstallMdmChangeEvent(aaModemChg);
aaInstallPeriodicEvent(EvPeriodic);
aaEnPeriodicEvent(
TRUE
);
.
.
.
}
#pragma check_stack(off) /* Microsoft C only */
void ExRxData(int Dev) /* receive event function */
{
int Count;
Count = aaGetRxCount(Dev); /* get number bytes available */
if(Count >
BUF_SIZE
)
Count =
BUF_SIZE
);
GetRxData(Dev,Count); /* application function to read the data */
}
void EvMdmChg(int Dev,unsigned char MdmChange,unsigned char MdmState)
{
if(MdmChange &
COM_MDM_CD
) /*
CD
changed */
{
CD
_Change[Dev]++; /* indicate change occurred */
}
ModemState[Dev] = MdmState; /* save current state of modem inputs */
}
void EvPeriodic(void) /* periodic event function */
{
int Dev;
for(Dev = FirstDev;Dev <= MaxDev;Dev++) /* check all devs for Tx data*/
{
SendTxData(Dev); /* application function to transmit data */
}
}
#pragma check_stack(on) /* Microsoft C only */
Each of the previously described event functions require different parameters.
For example, the receive data event function only passes a device number to
the application, whereas the modem change event function passes a device, a
modem state, and a modem change parameter to the application’s event
function.
These parameters are described in Appendix A under the function names
prefixed with Ev.
The periodic event is different from the other events in that it occurs on regular
intervals regardless of what is occurring on the controller. One use for the
periodic event function is to allow the application to write data to devices in the
background. See Subsection 2.13 for more information.
Warnings: The event functions you write for your applications are actually
executing during a system interrupt service routine (
ISR
). It is very
important that you keep these event functions as short as possible.
Also, there are many standard C library functions that do not work
within an
ISR
, such as printf(). Using these functions can cause
unpredictable results and can even hang your system.
If using the Microsoft C compiler, stack checking must be disabled