Syntax, Example, Strncpy( ) function – Echelon Neuron C User Manual
Page 161
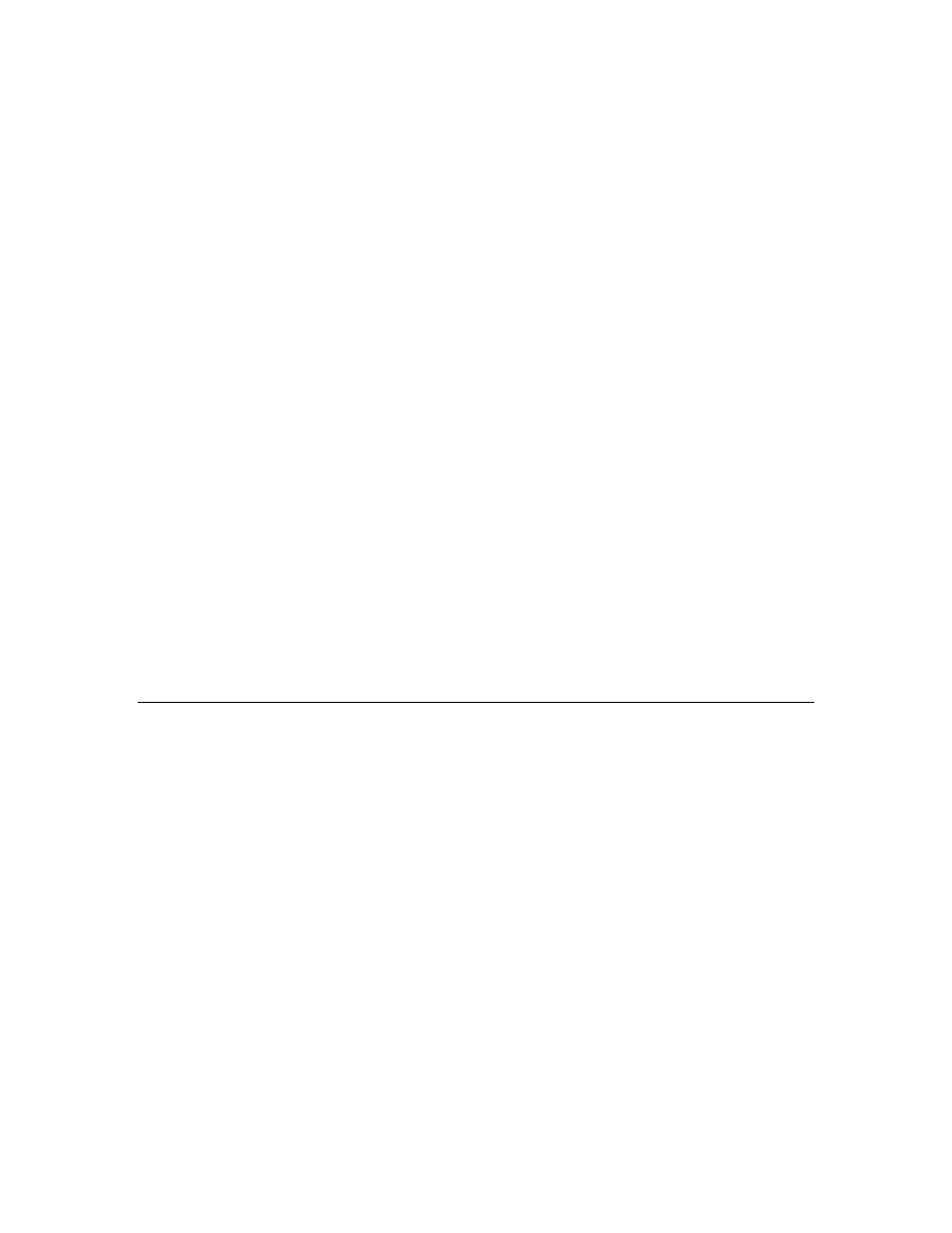
Neuron C Reference Guide
141
When a mismatch occurs, the characters from both strings at the mismatch are
compared. If the first string’s character is greater using an unsigned comparison,
the return value is positive. If the second string’s character is greater, the return
value is negative. The terminating NUL character is compared just as any other
character. See also strcat( ), strchr( ), strcmp( ), strcpy( ), strlen( ), strncat( ),
strncpy( ), and strrchr( ).
Syntax
#include
int strncmp (const unsigned char *
s1
, const unsigned char *
s2
,
unsigned
long
len
);
Example
#include
void f(void)
{
int
val;
char s1[20], s2[20];
val = strncmp(s1, s2, 10); // Compare first 10 chars
if (!val) {
// Strings are equal within the first 10 characters
} else if (val < 0) {
// String s1 is less than s2 (first 10 chars)
} else {
// String s1 is greater than s2 (first 10 chars)
}
}
strncpy( )
Function
The strncpy( ) function copies the string pointed to by the
src
parameter into the
string buffer pointed to by the
dest
parameter. The copy ends either when the
terminating NUL ('\0') character is copied or when
len
characters have been
copied, whichever comes first.
The function returns
dest
.
If the copy is terminated by the length, a NUL character is
not
added to the end
of the destination string. See also strcat( ), strchr( ), strcmp( ), strcpy( ), strlen( ),
strncat( ), strncmp( ), and strrchr( ).
This function cannot be used to copy overlapping areas of memory, or to write
into EEPROM memory or network variables.
Syntax
#include
char *strncpy (char *
dest
, const char *
src
, unsigned long
len
);