Apple WebObjects 3.5 User Manual
Page 145
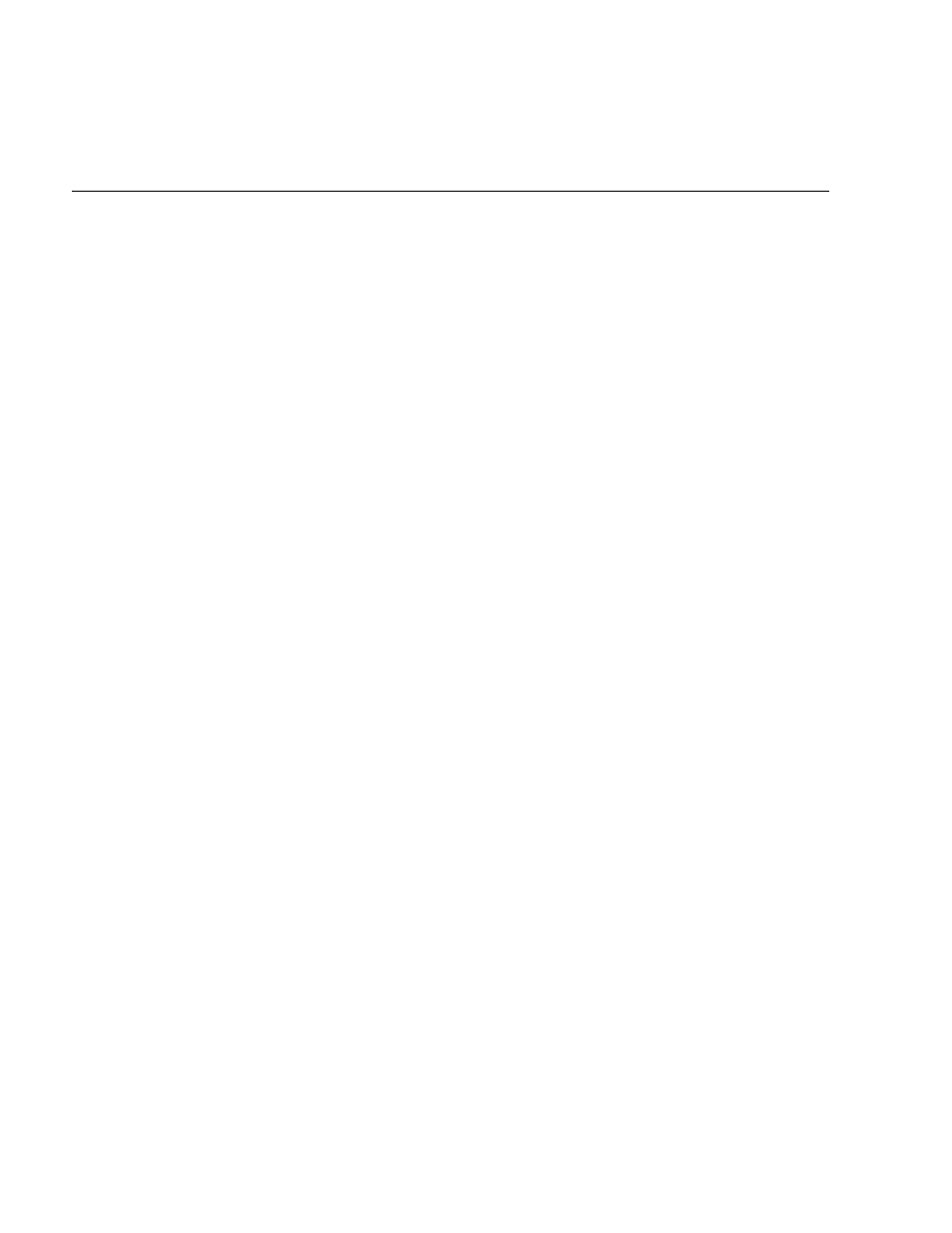
When You Have an Applet’s Source Code
145
1. In Project Builder, add the ClientSideJava subproject to your project.
To do so, double-click the word “Subprojects” in the browser and then
choose
ClientSideJava.subproj
in the Open panel.
When you build your project, Project Builder builds both the
individual Java
.class
files and a
.jar
file containing the entire
ClientSideJava subproject. This way, you have the option of using
WOApplet’s
archive
binding for browsers that support
.jar
files.
2. Add your class to the ClientSideJava subproject. Double-click Classes
in the subproject and then choose your
.java
file in the Open panel.
3. In the class declaration, insert the “implements
SimpleAssociationDestination” clause.
public class MyApplet extends Applet implements
SimpleAssociationDestination {
....
}
4. Implement the
keys
method to return a list (Vector) of state keys
managed by the applet.
public Vector keys() {
Vector keys = new Vector(1);
keys.addElement("title");
return keys;
}
5. Implement the
takeValueForKey
and
valueForKey
methods to set and get the
values of keys.
synchronized public Object valueForKey(String key) {
if (key.equals("title")) {
return this.getLabel();
}
}
synchronized public void takeValueForKey(Object value, String key) {
if (key.equals("title")) {
if ((value != null) && !(value instanceof String) {
System.out.println("Object value of wrong type set for key
'title'. Value must be a String.");
} else {
this.setLabel(((value == null) ? "" : (String)value));
}
}
You should be able to access the keys directly or, ideally, through
accessor methods (in this example,
getLabel
and
setLabel
). It is a good idea
to use the
synchronized
modifier with
takeValueForKey
and
valueForKey
because
these methods can be invoked from other threads to read or set data.