Sensoray 518 User Manual
Page 15
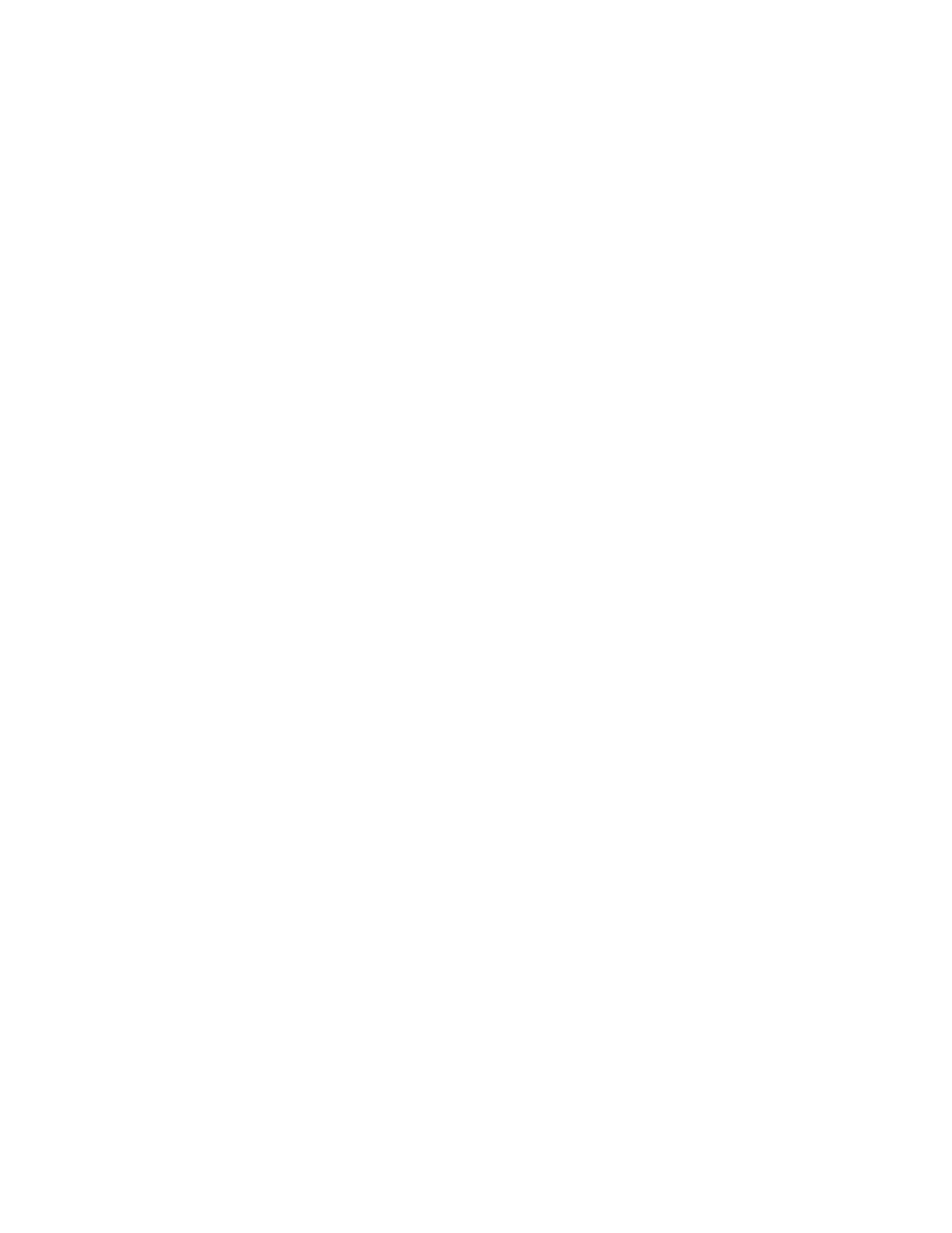
14
‘ ******************* QUICKBASIC DRIVERS *********************
‘ This subprogram handshakes a byte to the 518 command register:
SUB Send518Byte (BasePort As Integer, CommandByte As Integer)
DO : LOOP WHILE (INP(BasePort + 1) AND &H80) = 0
‘wait for CRMT
OUT (BasePort, CommandByte)
‘send the byte
END SUB
‘ This function handshakes a byte from the 518 data register:
FUNCTION Read518Byte% (BasePort As Integer)
DO : LOOP WHILE (INP(BasePort + 1) AND &H40) = 0 ‘wait for DAV
Read518Byte = INP(BasePort) ‘read the byte
END FUNCTION
You may find the following routines useful if you are writing a QuickBasic application. The first
procedure reads a 16-bit value from the coprocessor, adjusting the sign if necessary. The second
procedure sends a 16-bit value to the coprocessor. Note that these two routines make calls to the
QuickBasic procedures listed previously.
‘This function reads a 16-bit integer value from the 518.
FUNCTION Read518Word% (BasePort%)
Lval& = Read518Byte%(BasePort%)
‘read high data byte from 518
Lval& = Lval& * 256 + Read518Byte%(BasePort%)
‘read & concatenate low byte
IF Lval& > 32767 THEN Lval& = Lval& - 65536&
‘adjust sign, if necessary
Read518Word% = Lval&
‘exit with value
END FUNCTION
‘This subprogram writes a 16-bit integer value to the 518.
SUB Send518Word (BasePort%, Value%)
CALL Send518Byte (BasePort%, (Value% \ 256) AND &HFF) ‘send high byte to 518
CALL Send518Byte (BasePort%, Value% AND &HFF) ‘send low byte to 518
END SUB