Sample low-level drivers – Sensoray 518 User Manual
Page 14
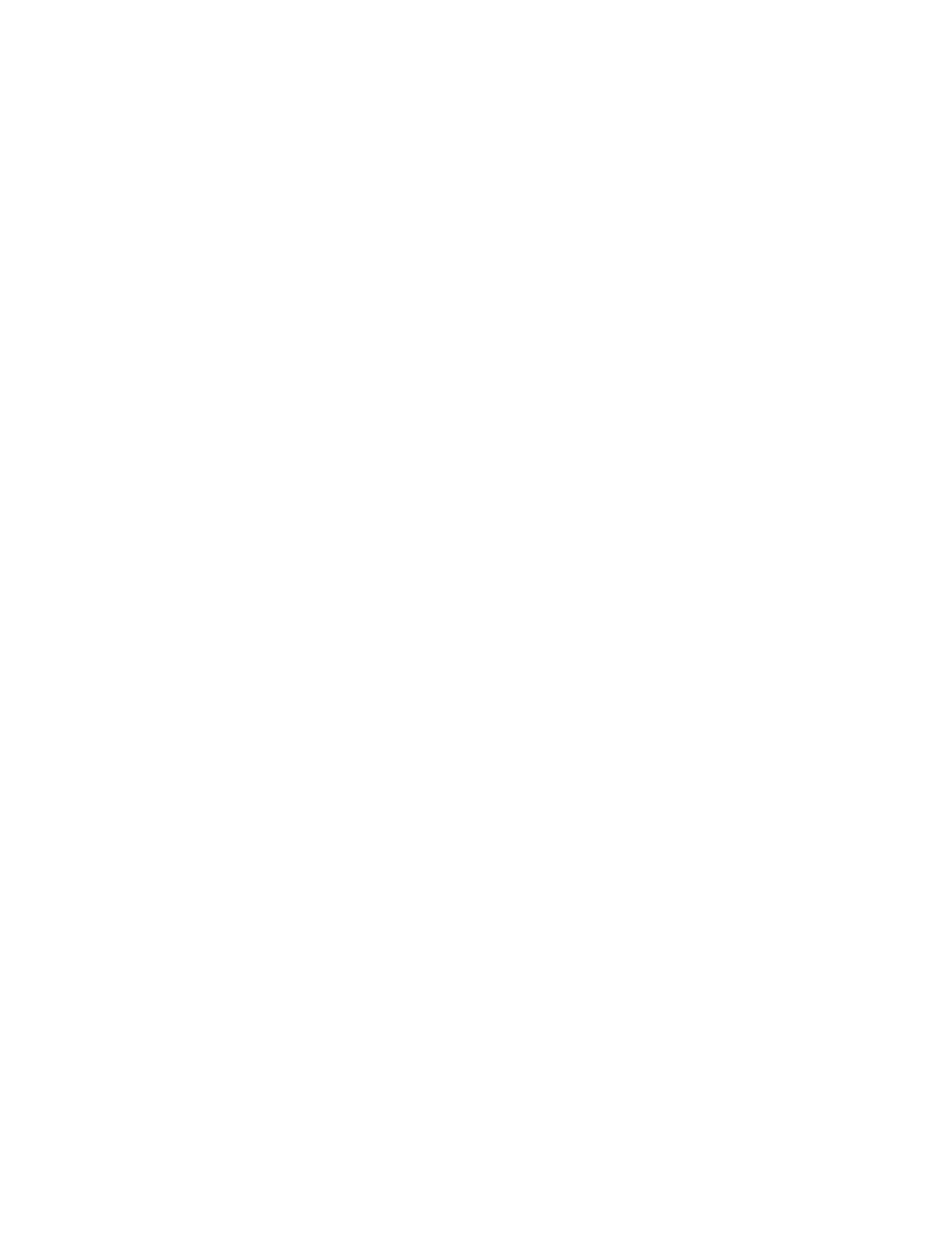
13
Sample Low-level Drivers
We recommend that you incorporate procedures in your application software that will conceal
the handshake protocol from higher host software levels. This will serve to simplify your
programming requirements as well as reduce software maintenance costs.
This section contains sample software drivers that implement the required handshake protocol. In
addition, the QuickBasic routines outlined below are referenced throughout this chapter in
programming examples. Feel free to plagiarize and adapt these routines for your application.
; *************** 80x86 ASSEMBLY LANGUAGE DRIVERS ******************
; This procedure sends a command byte to the 518.
; Entry: DX points to 518 base port address.
; AL contains command byte to send to 518.
XMIT: PUSH AX ; SAVE COMMAND BYTE TO BE SENT TO 518
INC DX ; POINT DX TO 518 STATUS PORT
XLOOP: IN AL,DX ; READ STATUS PORT
TEST AL,80H ; AND MASK “COMMAND REGISTER EMPTY” BIT
JE XLOOP ; LOOP BACK UNTIL REGISTER IS EMPTY
DEC DX ; POINT DX TO 518 COMMAND/DATA PORT
POP AX ; RESTORE COMMAND BYTE
OUT DX,AL ; AND WRITE IT INTO 518 COMMAND REGISTER
RET ; EXIT COMMAND REGISTER DRIVER ;
; This procedure reads a data byte from the 518.
; Entry: DX points to 518 base port address.
; Exit: AL contains data byte read from 518.
RCV: INC DX ; POINT DX TO 518 STATUS PORT
RLOOP: IN AL,DX ; READ STATUS PORT
TEST AL,40H ; AND MASK “DATA AVAILABLE” BIT
JE RLOOP ; LOOP BACK UNTIL DATA IS AVAILABLE
DEC DX ; POINT DX TO 518 COMMAND/DATA PORT
IN AL,DX ; READ DATA FROM 518 DATA REGISTER
RET ; EXIT DATA REGISTER DRIVER