HP Integrity NonStop J-Series User Manual
Page 90
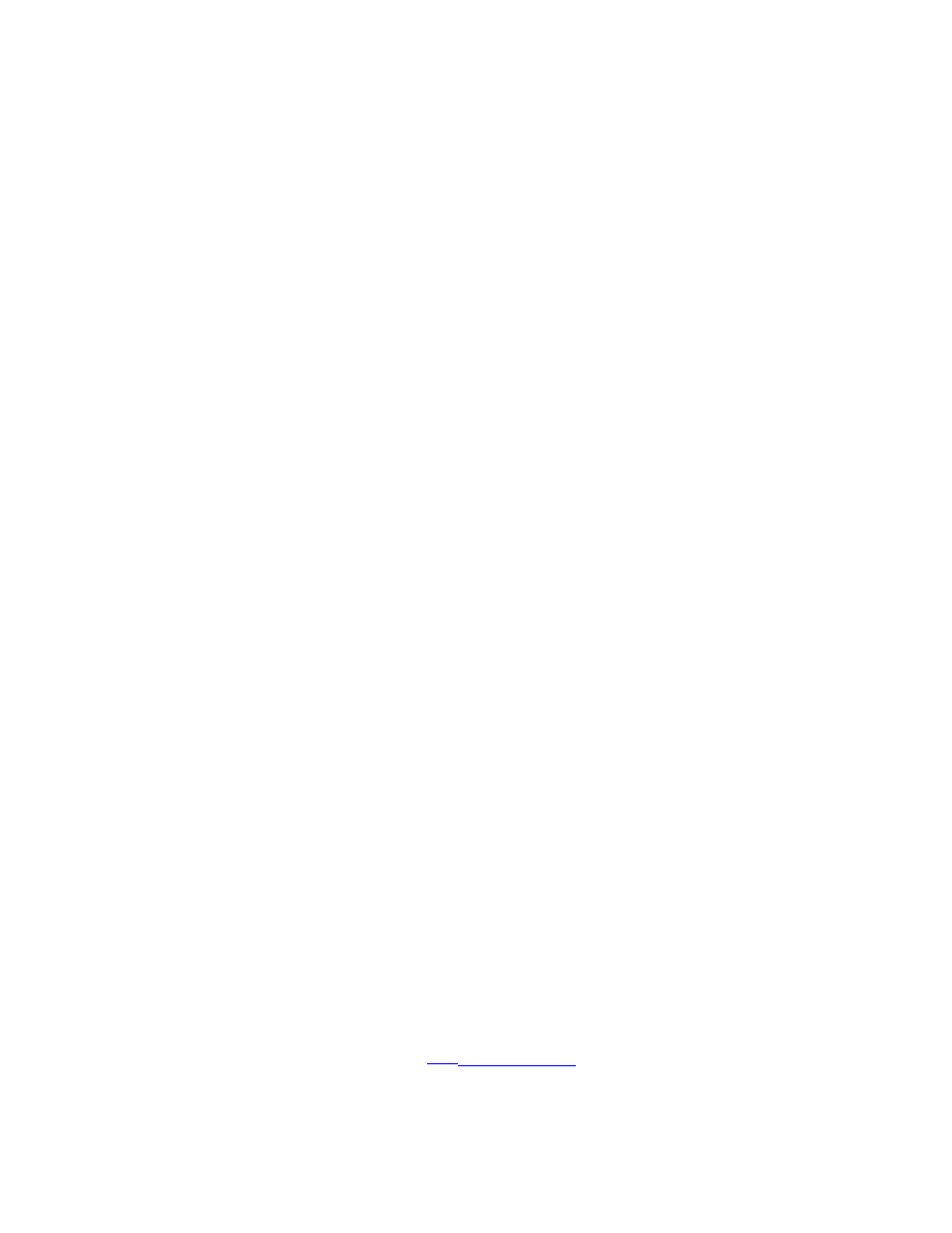
#include
struct DiskNode { // 2
int data; // 3
Rwoffset nextNode; // 4
};
main(){
RWFileManager fm("linklist.dat"); // 5
// Allocate space for offset to start of the linked list:
fm.allocate(sizeof(RWoffset)); // 6
// Allocate space for the first link:
RWoffset thisNode = fm.allocate(sizeof(DiskNode)); // 7
fm.SeekTo(fm.start()); // 8
fm.Write(thisNode); // 9
DiskNode n;
int temp;
RWoffset lastNode;
cout << "Input a series of integers, ";
cout << "then EOF to end:\n";
while (cin >> temp) { // 10
n.data = temp;
n.nextNode = fm.allocate(sizeof(DiskNode)); // 11
fm.SeekTo(thisNode); // 12
fm.Write(n.data); // 13
fm.Write(n.nextNode);
lastNode = thisNode; // 14
thisNode = n.nextNode;
}
fm.deallocate(n.nextNode); // 15
n.nextNode = RWNIL; // 16
fm.SeekTo(lastNode);
fm.Write(n.data);
fm.Write(n.nextNode);
return 0;
} // 17
Here's a line-by-line description of the program:
//1
Include the declarations for the class
RWFileManager
.
//2
Struct DiskNode is a link in the linked-list. It contains:
//3
the data (an int), and:
//4
the offset to the next link. RWoffset is typically typedef'd to a long int.