HP Integrity NonStop J-Series User Manual
Page 278
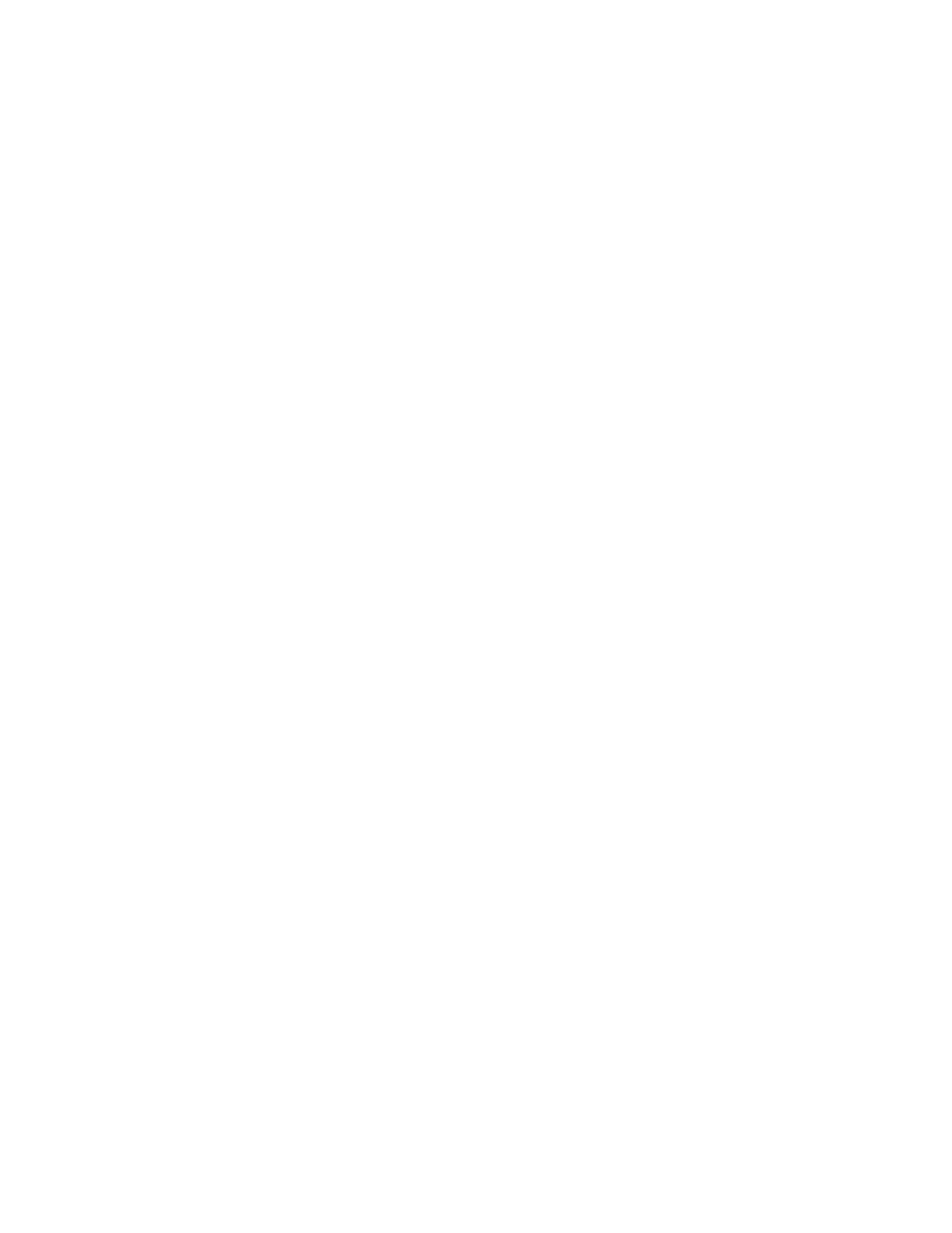
Here is a more sophisticated example of a class that uses these features:
#include
#include
#include
class Tangle : public RWCollectable
{
public:
RWDECLARE_COLLECTABLE(Tangle)
Tangle* nextTangle;
int someData;
Tangle(Tangle* t = 0, int dat = 0){nextTangle=t; someData=dat;}
virtual void saveGuts(RWFile&) const;
virtual void restoreGuts(RWFile&);
};
void Tangle::saveGuts(RWFile& file) const{
RWCollectable::saveGuts(file); // Save the base class
file.Write(someData); // Save internals
file << nextTangle; // Save the next link
}
void Tangle::restoreGuts(RWFile& file){
RWCollectable::restoreGuts(file); // Restore the base class
file.Read(someData); // Restore internals
file >> nextTangle; // Restore the next link
}
// Checks the integrity of a null terminated list with head "p":
void checkList(Tangle* p){
int i=0;
while (p)
{
assert(p->someData==i);