Apply functions – HP Integrity NonStop J-Series User Manual
Page 147
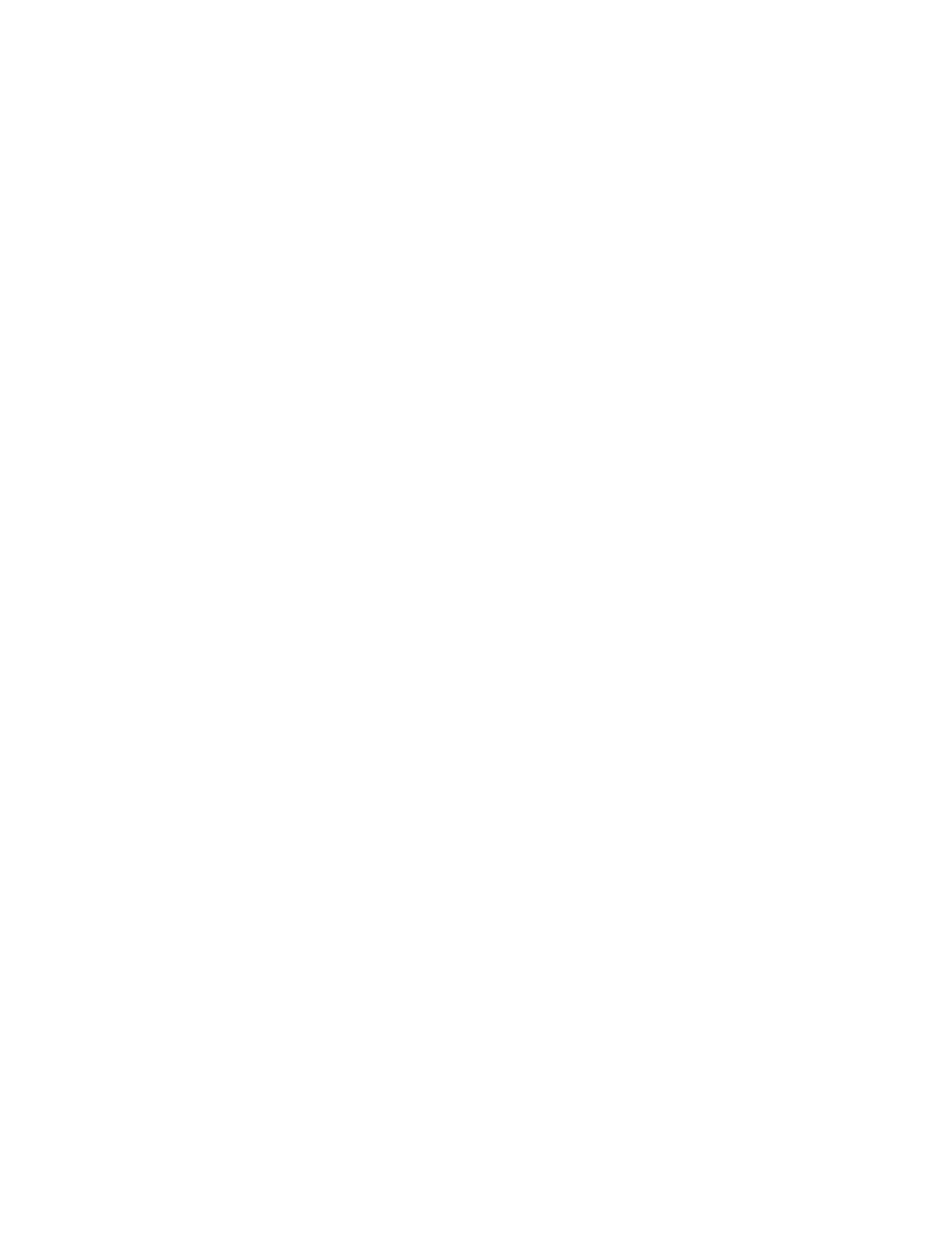
//9
Here the member function contains() is called, using the tester function. The second
argument of contains(), a pointer to the variable aValue, will appear as the second
argument of the tester function. The function contains() traverses the entire stack,
calling the tester function for each item in turn, and waiting for the tester function to
signal a match. If it does, contains() returns TRUE; otherwise, FALSE.
Note that the second argument of the tester function does not necessarily have to be of the same
type as the members of the collection, although it is in the example above. In the following
example, the argument and members of the collection are of different types:
#include
#include
class Foo {
public:
int data;
Foo(int i) {data = i;}
};
declare(RWGStack, Foo) // A stack of pointers to Foos
RWBoolean anotherTesterFunction(const Foo* fp, const void* a)
{ return fp->data == *(const int*)a;
}
main(){
RWGStack(Foo) gs;
gs.push(new Foo(1));
gs.push(new Foo(2));
gs.push(new Foo(3));
gs.push(new Foo(4));
int aValue = 2;
if ( gs.contains(anotherTesterFunction, &aValue) )
cout << "Yup.\n";
else
cout << "Nope.\n";
while(!gs.isEmpty())
delete gs.pop();
return 0;
}
In this example, a stack of (pointers to) Foos is declared and used, while the variable being
passed as the second argument to the tester function is still a const int*. The tester function must
take the different types into account.