Rwdeclare_persistable to your header file – HP Integrity NonStop J-Series User Manual
Page 184
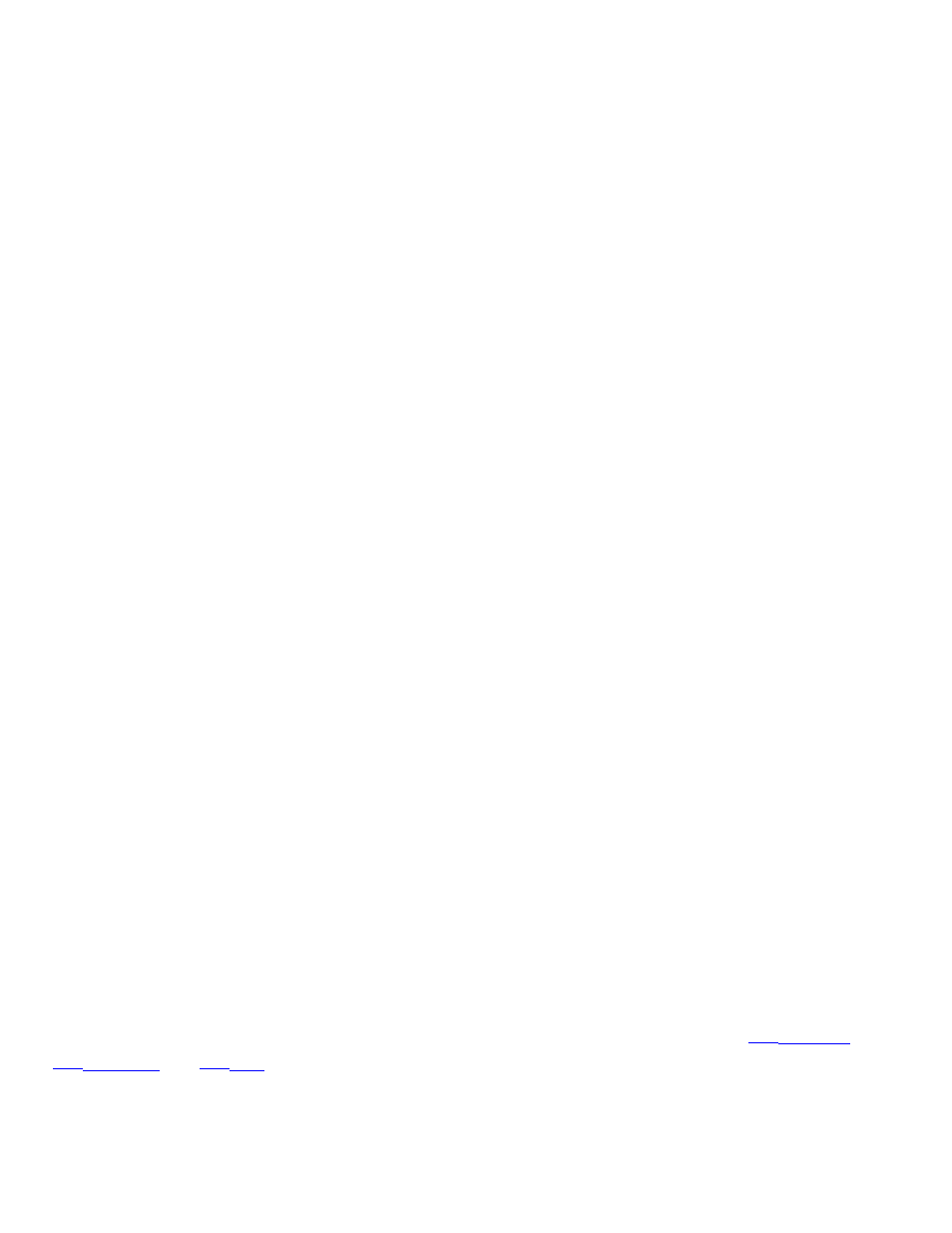
friend void rwSaveGuts(RWvostream&, const Friendly&);
friend void rwRestoreGuts(RWFile&, Friendly&);
friend void rwSaveGuts(RWFile&, const Friendly&);
friend void rwRestoreGuts(RWvistream&, Friendly&);
//...
};
Or your class could have accessor functions to the restricted but necessary members:
class Accessible {
public:
int secret(){return secret_}
void secret(const int s){secret_ = s}
//...
private:
int secret_;
};
If you can't change the source code for the class to which you want to add isomorphic persistence,
then you could consider deriving a new class that provides access via public methods or friendship:
class Unfriendly{
protected:
int secret_;
// ...
};
class Friendlier : public Unfriendly {
public:
int secret(){return secret_}
void secret(const int s){secret_ = s}
//...
};
If you can't change the source code for a class, you will be unable to isomorphically persist private
members of that class. But remember: you only need access to the data necessary to recreate the class
object, not to all the members of the class. For example, if your class has a private cache that is
created at run time, you probably don't need to save and restore the cache. Thus, even though that
cache is private, you don't need access to it in order to persist the class object.
Add RWDECLARE_PERSISTABLE to Your Header File
Once you have determined that all necessary class data is accessible, you must add declaration
statements to your header files. These statements declare the global functions operator<< and
operator>> for your class. The global functions permit storage to and retrieval from
RWvistream
,
RWvostream
and
RWFile
.
Tools.h++ provides several macros that make adding these declarations easy. The macro you choose
depends upon whether your class is templatized or not, and if it is templatized, how many templatized
parameters it has.