HP Integrity NonStop J-Series User Manual
Page 132
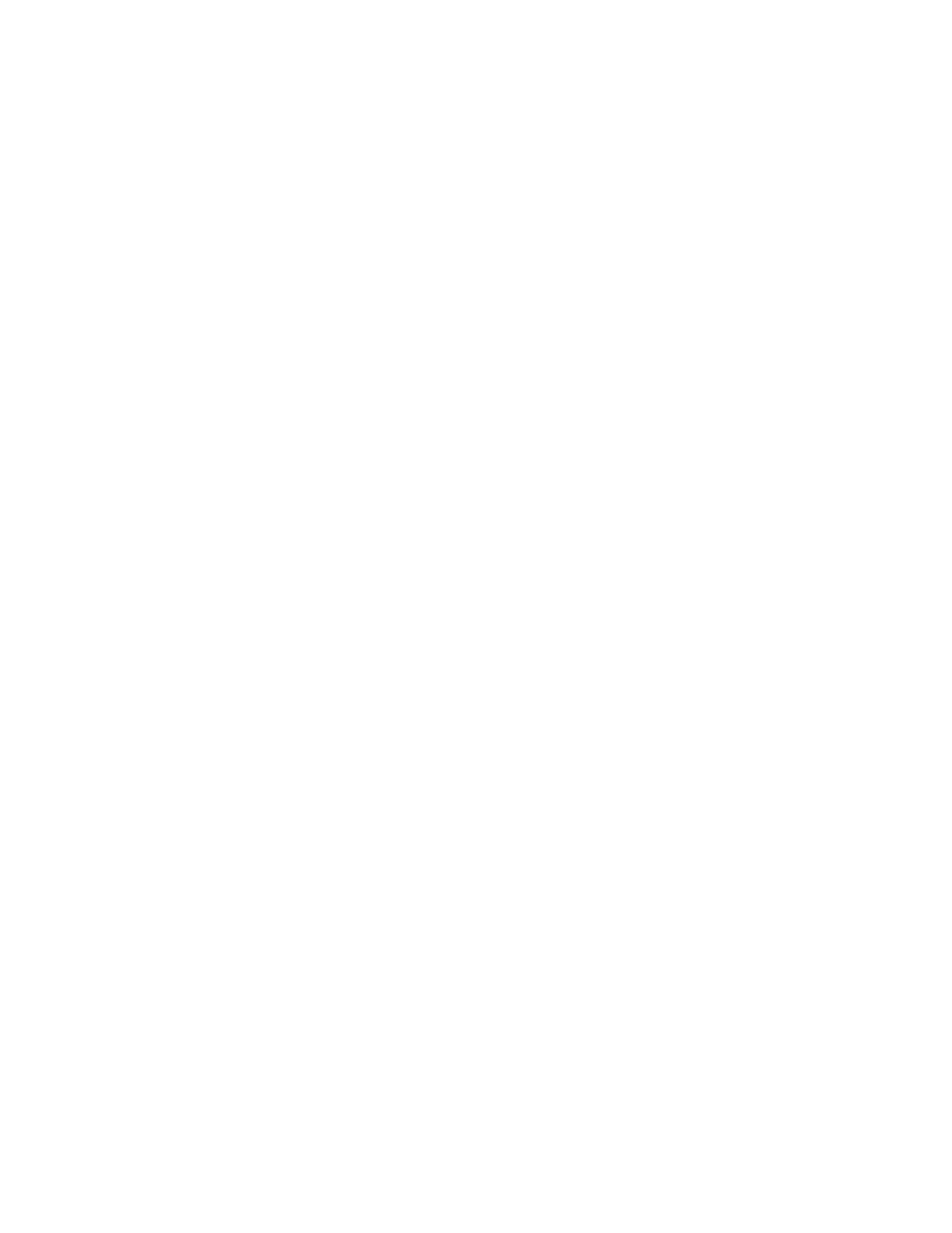
// generate the next Card
Card operator()() {
rankIdx = (rankIdx + 1) % 13;
if (rankIdx == 0)
// cycled through ranks, move on to next suit:
suitIdx = (suitIdx + 1) % 4;
return Card(Ranks[rankIdx], Suits[suitIdx]);
}
};
const char DeckGen::Suits[4] = {'S', 'H', 'D', 'C' };
const char DeckGen::Ranks[13] = {'A', '2', '3', '4',
'5', '6', '7', '8',
'9', 'T', 'J', 'Q', 'K' };
int main(){
// Tools.h++ collection:
RWTValOrderedVector
RWTValOrderedVector
Card aceOfSpades('A','S');
Card firstCard;
// Use standard library algorithm to generate deck:
generate_n(back_inserter(deck.std()), 52, DeckGen());
cout << endl << "The deck has been created" << endl;
// Use Tools.h++ member function to find card:
pos = deck.index(aceOfSpades);
cout << "The Ace of Spades is at position " << pos+1 << endl;
// Use standard library algorithm to shuffle deck:
random_shuffle(deck.begin(), deck.end());
cout << endl << "The deck has been shuffled" << endl;
// Use Tools.h++ member functions:
pos = deck.index(aceOfSpades);
firstCard = deck.first();
cout << "Now the Ace of Spades is at position " << pos+1
<< endl << "and the first card is " << firstCard << endl;
}
/* Output (will vary because of the shuffle):