HP Integrity NonStop J-Series User Manual
Page 81
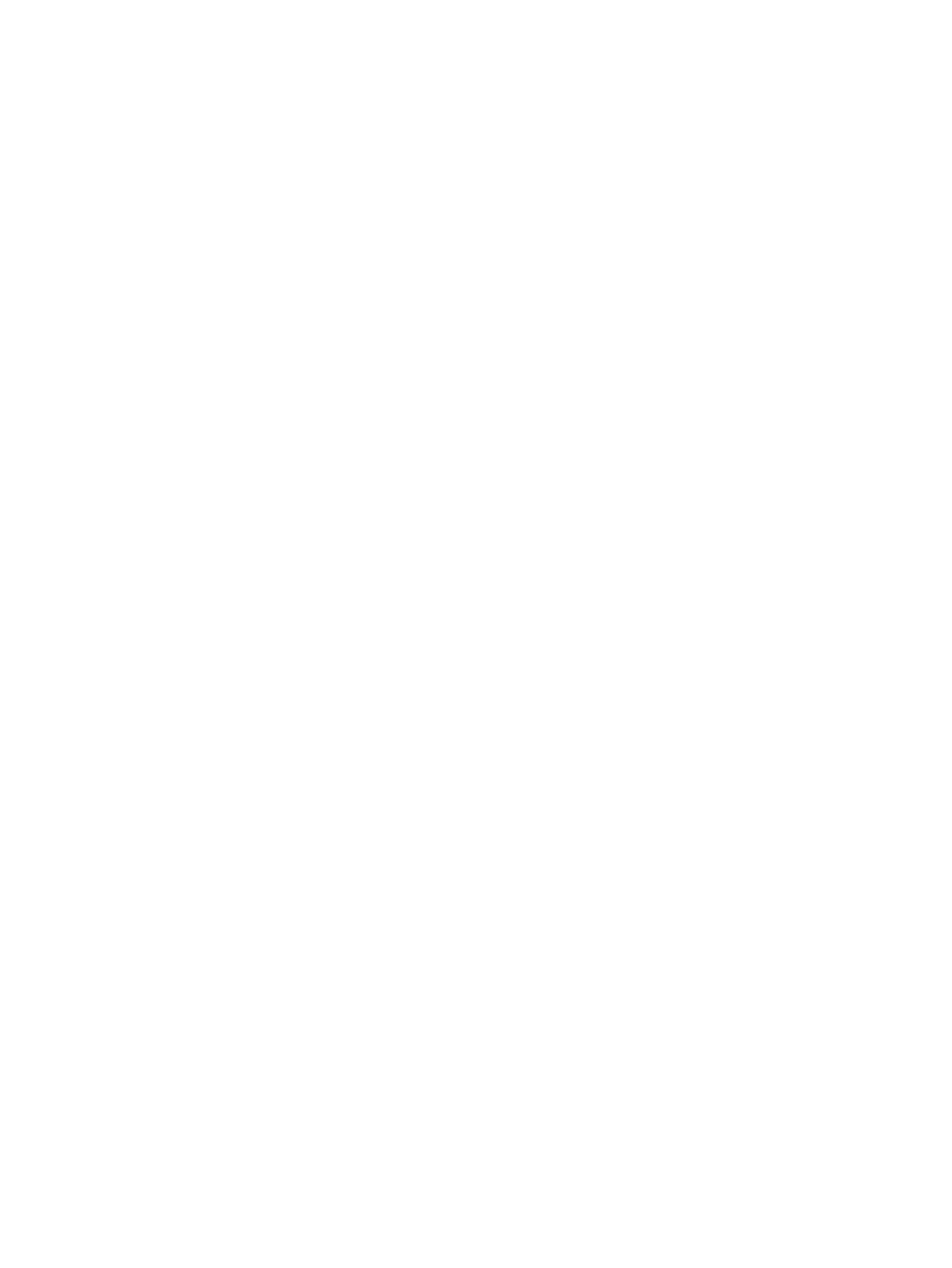
2.
Modify the EmployeeInfo.java file as follows:
package com.hp.empinfo.service;
import com.hp.empinfo.domain.Employee;
public interface EmployeeInfo {
public Employee getEmployee(int empId);
}.
This creates the RMI service interface which you must implement as described in the subsequent
section.
Creating Implementation Class of RMI service Interface
1.
Create a com.hp.empinfo.service package and an EmployeeInfoImpl class under
it, as described in
“Creating the Controller for EmpInfo” (page 54)
.
2.
Modify the EmployeeInfoImpl.java class as follows:
package com.hp.empinfo.service;
import java.sql.SQLException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.hp.empinfo.domain.Employee;
public class EmployeeInfoImpl implements EmployeeInfo {
private EmployeeDao empdaoBean; ;
public EmployeeDao getEmpdaoBean() {
return empdaoBean;
}
public void setEmpdaoBean(EmployeeDao empdaoBean) {
this.empdaoBean = empdaoBean;
}
public static String [] configFileNames = new String [] {"applicationContext.xml","clientContext.xml"};
private static ApplicationContext applicationContext = null;
public Employee getEmployee(int empId) {
Employee detail = null;
applicationContext = new ClassPathXmlApplicationContext(configFileNames);
EmployeeDao empDao = (EmployeeDao)applicationContext.getBean("empdao");
try {
detail = empDao.getDetail(empId);
} catch (SQLException e) {
e.printStackTrace();
}
return detail;
}
}
Modifying the applicationContext.xml file
You must modify the applicationContext.xml file to add the Spring RMI bean. To achieve
this, add the following code in the file:
Getting Started with Spring
81