Applied Motion SV7-Q-EE User Manual
Page 17
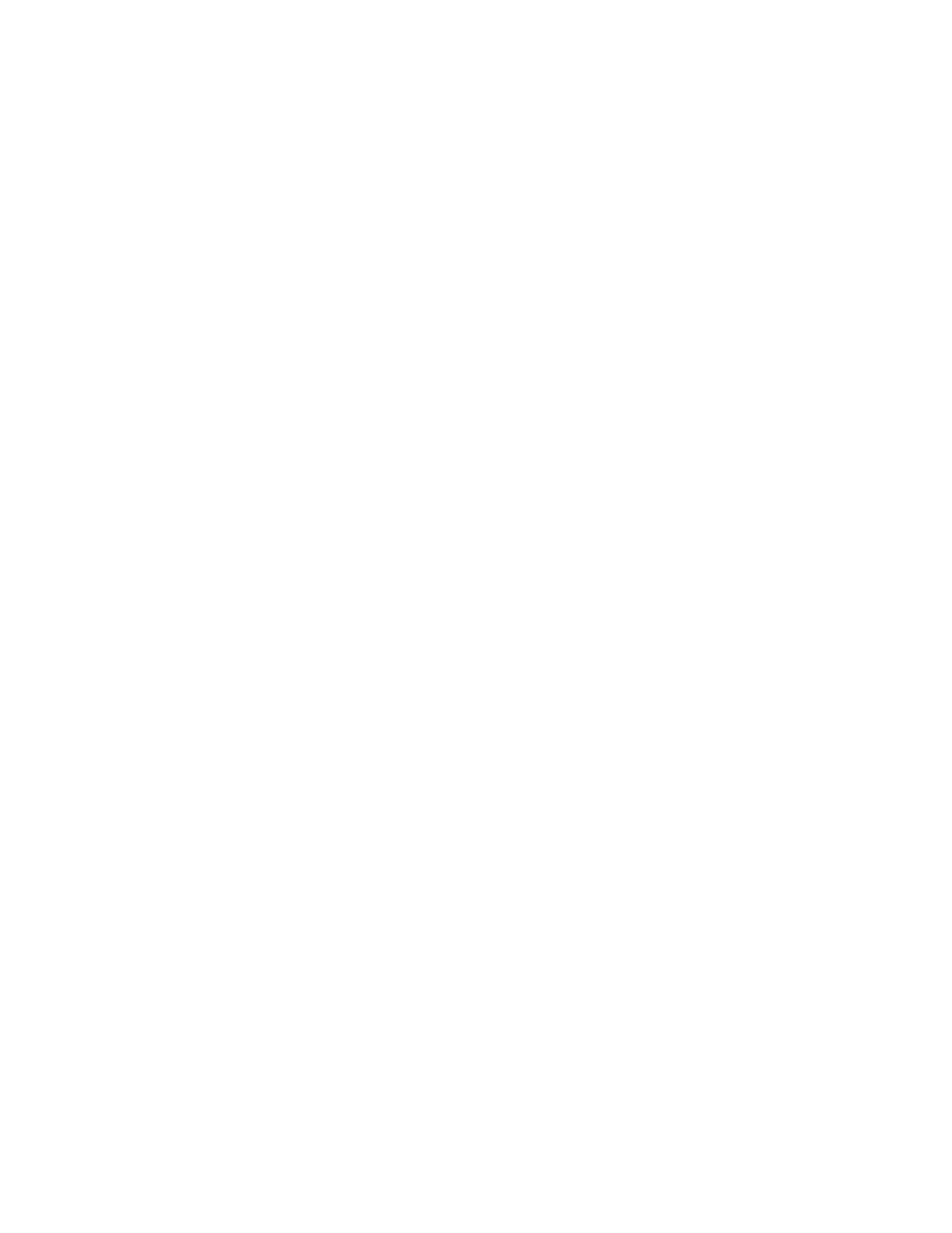
6/26/2010
920‐0032a3 eSCL Communication Reference Manual
Page 17
Polling is easier to code but less efficient because you must either sit in a loop waiting for an expected
response or run a timer to periodically check for data coming in. Since the choice depends on your
programming style and the requirements of your application, we present both techniques.
Polling for an incoming packet
The same UdpClient object that you use to send packets can be used to retrieve incoming responses from the
drive. The Available property will be greater than zero if a packet has been received. To retrieve a packet,
assign the Receive property to a Byte array. You must create an IPEndPoint object in order to use the Receive
property.
private
void
UDPpoll()
{
// you can call this from a timer event or a loop
if
(udpClient.Available > 0)
// is there a packet ready?
{
IPEndPoint
RemoteIpEndPoint =
new
IPEndPoint
(
IPAddress
.Any, 0);
try
{
// Get the received packet. Receive method blocks
// until a message returns on this socket from a remote host,
// so always check .Available to see if a packet is ready.
Byte
[] receiveBytes = udpClient.Receive(
ref
RemoteIpEndPoint);
// strip opcode
Byte
[] SCLstring =
new
byte
[receiveBytes.Length - 2];
for
(
int
i = 0; i < SCLstring.Length; i++)
SCLstring[i]
=
receiveBytes[i
+
2];
string
returnData =
Encoding
.ASCII.GetString(SCLstring);
AddToHistory(returnData);
}
catch
(
Exception
ex)
{
// put your error handler here
Console
.WriteLine(ex.ToString());
}
}
}
Creating a receive event using a call back function
First, create a function to handle incoming packets. This function must contain two local objects: a UdpClient
and an IPEndPoint. The call back function will be passed an IAsyncResult object that contains a reference to
the UDP connection. The local IPEndPoint object is passed to the UDPClient’s EndReceive property to retrieve
the packet.
public
void
ReceiveCallback(
IAsyncResult
ar)
{
int
opcode;
UdpClient
u = (
UdpClient
)((
UdpState
)(ar.AsyncState)).u;
IPEndPoint
e = (
IPEndPoint
)((
UdpState
)(ar.AsyncState)).e;
Byte
[] receiveBytes = u.EndReceive(ar,
ref
e);
// get opcode
opcode = 256 * receiveBytes[0] + receiveBytes[1];
if
(opcode == 7)
// SCL response
{
string
receiveString =
Encoding
.ASCII.GetString(receiveBytes);