Basic programming – Xylem STORM 3 Basic Programming manual User Manual
Page 8
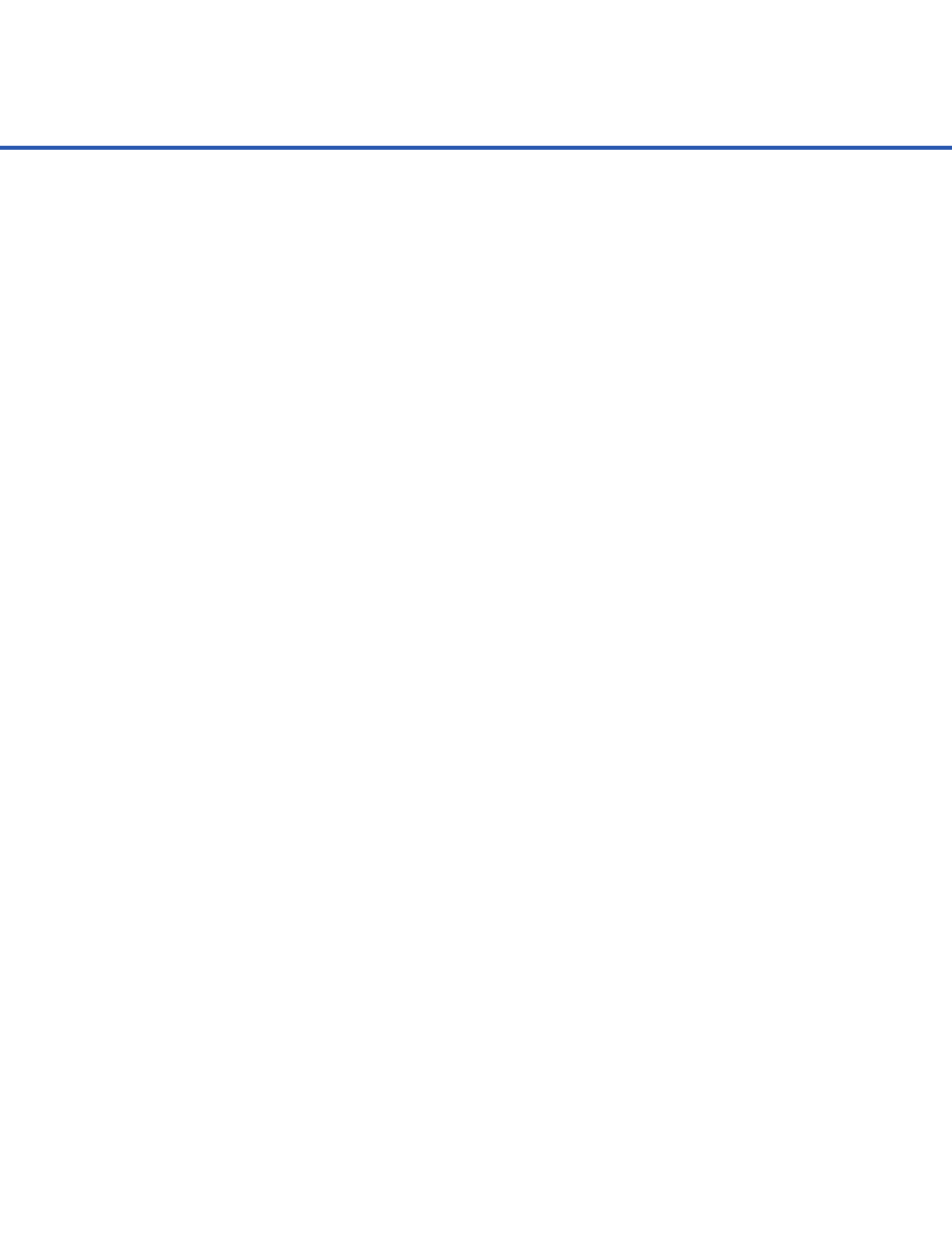
BASIC PROGRAMMING
Basic supports traditional control statements such as GOTO and GOSUB as well as single and
multi-line IF-THEN statements and SWITCH-CASE statements. GOTO and GOSUB can jump to
labels or line numbers within the code. Line numbers may be used in the Basic program, but they
are not required.
Loops are also allowed using FOR, WHILE, REPEAT, and DO statements. BREAK statements may be
used to leave any of the previously mentioned loops while the CONTINUE command can be used
to begin the next iteration of a loop
Control Statements and Loops
6
In order to ease the structure and flow of a Basic program, subroutines may be declared and used
to perform various operations. The separation of these subroutines allows a more procedural
approach to be taken within the code as well as simplify the reuse of segments of code in later
programs. These subroutines can accept multiple parameters, numbers or strings, and can
likewise return a number or a string, if desired. Subroutines are declared with the SUB command,
ended with the END SUB command, and return values using the RETURN command.
REM Calculate the Volume of a Rectangle
height = 3
width = 4
depth = 5
volume = calc_volume(height, width, depth) REM sets volume to 60
SUB calc_volume(h, w, d)
vol = h * w * d
RETURN vol
END SUB
Subroutines
Basic provides a comprehensive list of math functions including logarithmic and trigonometric
functions. All trigonometric functions use radians. The following functions are available: ABS,
ACOS, ASIN, ATAN, CEIL, COS, EXP, FLOOR, FRAC, H377C, H377F, INT, LN, LOG, MAX, MIN, MOD,
RND, SGN, SIN, SQR, SQRT, TAN, XOR. Constants EULERand PI are also available for use within
Basic programs.
Math Functions
Traditional arithmetic, comparison, and logical operators are available for use within Basic and are
noted below (ordered by precedence):
Arithmetic, Comparison and Logical Operators
Arithmetic:
^ (power)
- (unary minus)
* / % (integer modulus)
+ -
Comparison:
= ==
<><= >=
<> !=
Logical:
NOT
AND
OR