Xylem STORM 3 Basic Programming manual User Manual
Page 7
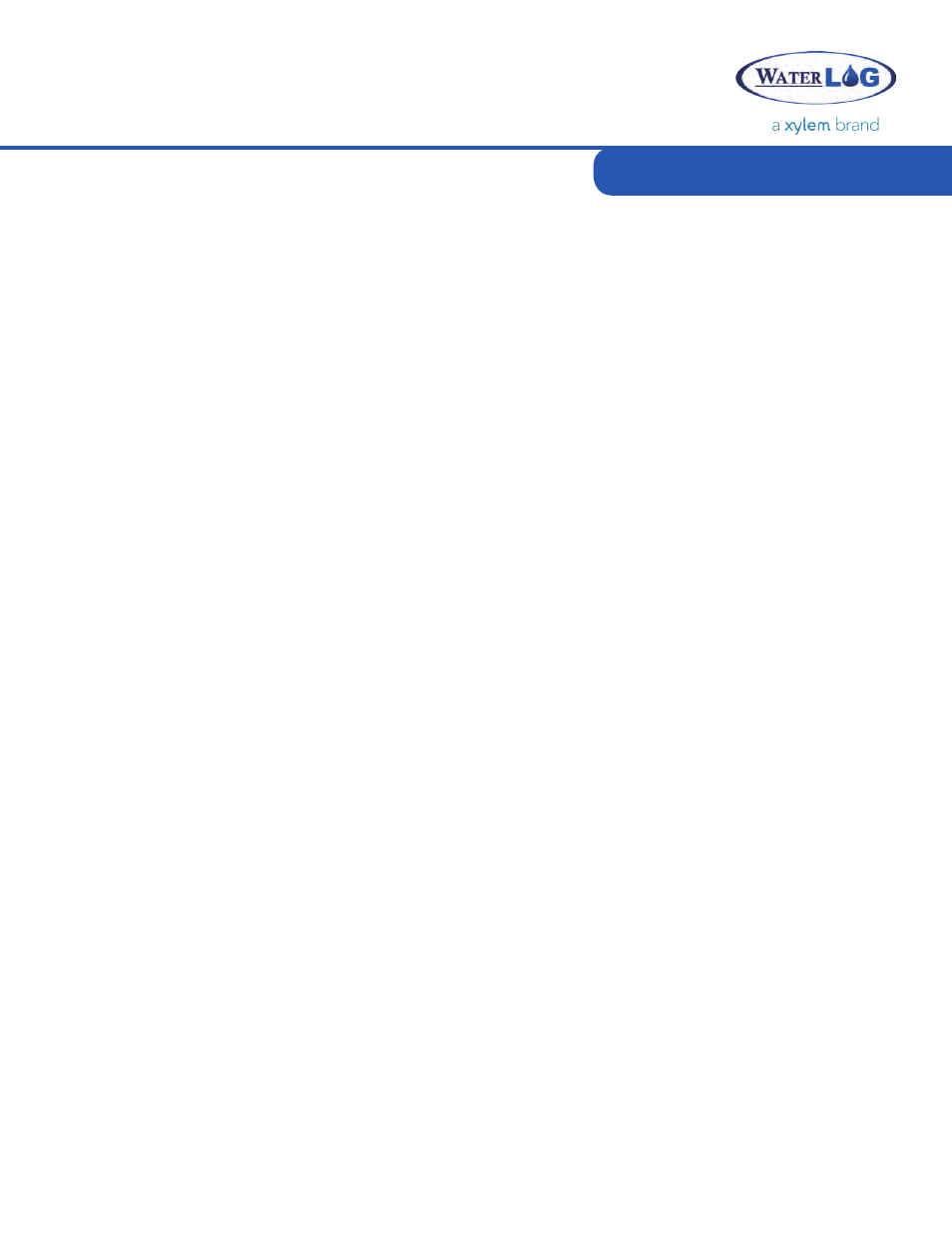
Basic Programming
5
Basic programming examples provided throughout the manual will follow a specific format. All
Basiccommands and functions will be capitalized while all variables and strings may contain both
uppercase and lowercase lettering. Though this format is used, the Basicinterpreter is not case-
sensitive with respect to commands and functions. Variables, however, are case-sensitive. In
other words, the following “PRINT”, “Print”, and “print” commands are all interpreted as valid PRINT
commands, whereas variables “var” and “Var” are interpreted as two different variables. Code
examples given in the manual will appear similar to the following:
PRINT “Hello World”
var =12345.67
Grammar and Examples
The REM statement (meaning remark) or single quote (‘) can be used to introduce a comment
that extends to the end of the line. Comments can exist anywhere and span a single line. Once
a comment has been started, the remainder of the line will also be considered a comment.
Comments are not necessary in a program (they are ignored by the interpreter), though can
provide helpful clarification when trying to understand. The following examples all represent valid
uses of comments:
REM This is a comment
‘ This is also a comment
PRINT “Hello World”REM This is a valid comment after a command
PRINT “Hello World” ‘ This is also a valid comment after a command
Comments
Unlike the case-insensitive nature of Basic statements, variables and strings are uniquely identified
by their names. Thus a variable named “var” is understood to be different than a similarly named
variable “Var”. Standard variables represent a number.
var = 15.2
REM sets var to 15.2
Var = 0 REM sets Var 0
String variables are also available and require a trailing “$”.
var$ = “Hello World” REM sets var$ to “Hello World”
Traditional variables do not need to be declared before their first use. Number variables are
initialized to zero (“0”) while string variables are initialized to an empty string (“”). Arrays, on the
other hand, need to be created first using the ARRAY command, prior to being used. Arrays
contain multiple number or string variables. Array contents are initialized to zeros or empty strings
depending on their declaration type.
REM create a single-dimension, two element number array
ARRAY myArray(2)
var$ = myArray(1), “ “, myArray(2) REM sets var to “0 0”
Variables, Strings and Arrays