Declarations – Crunch CRiSP File Editor 6 User Manual
Page 26
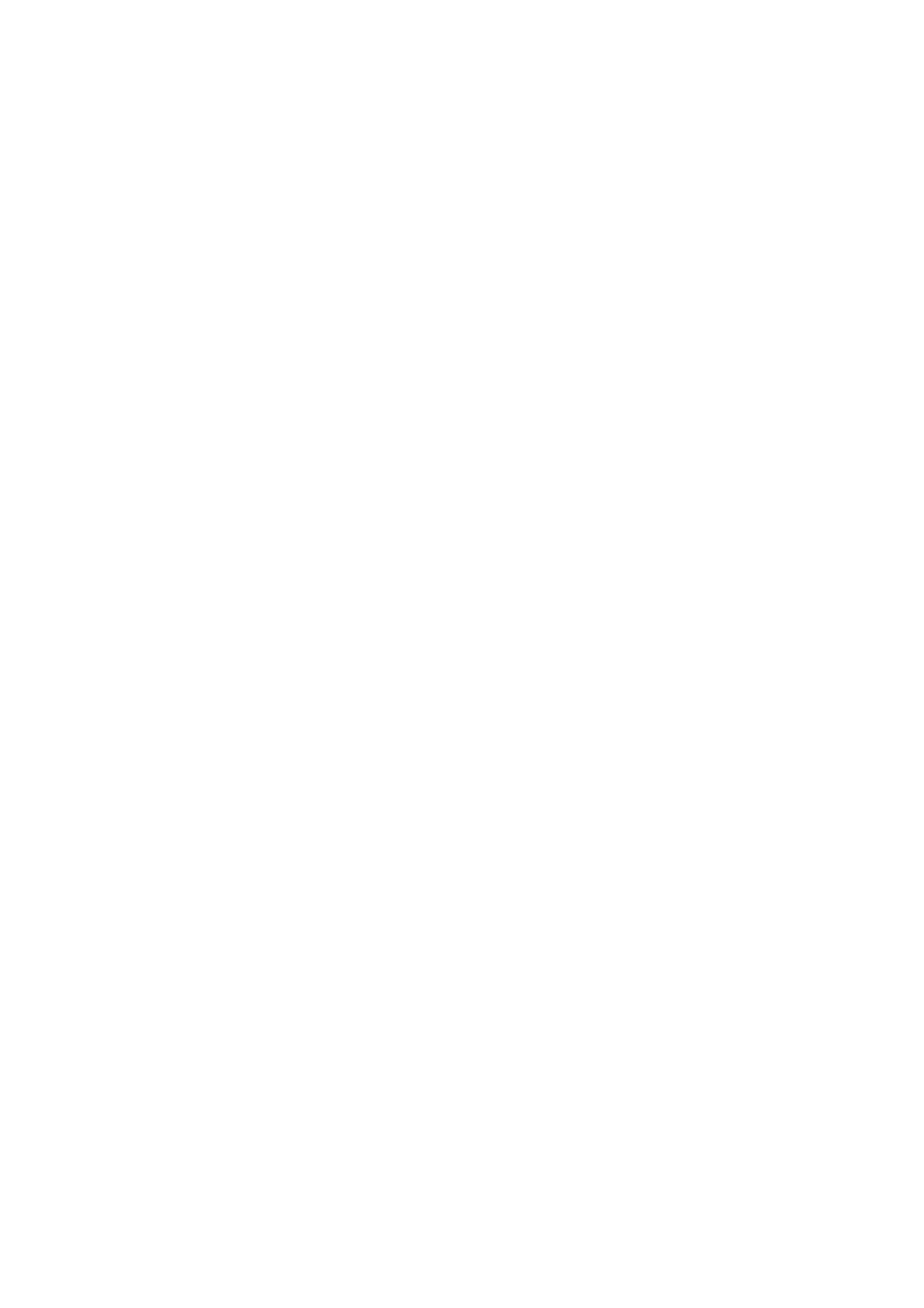
Page 26
→
Function definitions(pg. 27).
→
Expressions(pg. 27).
→
Loop constructs(pg. 28).
→
Conditional test - if(pg. 29).
→
Selection: switch(pg. 29).
{button See Also, Alink(crunch,,,)}
Declarations
There are two main types of declarations -- function definitions and data declarations. A function definition
defines the body of a function. A data declaration is used to declare global variables or specify prototypes. A
data declaration has the form:
[storage_class_specifier] [type_specifier] [declarator [= initializer]] ;
This is similar to C. The storage_class_specifier is used to identify how the variable is to be stored. The
currently supported and meaningful storage class specifiers are:
extern
The variable is defined somewhere else. References to the variable will be validated
against its type information, but no code will be generated to create the variable. This is
typically used to implement a forward reference mechanism.
static
When applied to a macro definition (function definition), the macro can only be invoked
from a macro defined within the same file. The macro function will not be visible or
accessible to any other macros or for use in callbacks. The use of the static keyword is
encouraged for all functions which are part of an implemented feature but of no use to
other functions, and also for functions which are not going to be called back, e.g. as a
result of a trigger, or keystroke.
When applied to a variable defined within a function, static has the same meaning as in
the C language, viz. the variable will maintain its value across function calls. A static
variable can be initialised, in which case the function will be initialised the first time the
function is called.
Type specifier should be one of the following:
int
Used to define a variable which will store a 32-bit integral value or to specify a function
which returns an int value.
float
double
Used to define a variable which will store a 64-bit floating point value or to specify a
function which returns a float value.
string
Defines a variable which can store an indefinite length string or a function which returns a
string value.
list
Defines a variable which can store a list value or a function which returns a list value.
declare
Defines a polymorphic variable which can store any data type, or a function which can
return any type.
void
Used to indicate a function which doesn't return a value.
A declarator is defined as one or more variable names, or function prototypes separated by commas.
An initializor is used to give a variable an initial value, similar to C. Initializors may be arbitrary expressions,
i.e. they are not limited to constant expressions, even for global variables. Lists may be initialised somewhat
similarly to C structure initialisors.
For example:
extern list fred;
int func (int, string, string);
int a, b = 1;